Advice on a better way to extend C++ STL container with user-defined methods(关于使用用户定义方法扩展 C++ STL 容器的更好方法的建议)
问题描述
我从 C++ STL 容器继承并添加了我自己的方法.其基本原理是,对于客户端来说,它看起来像一个常规列表,但具有可以轻松调用的特定于应用程序的方法.
I inherited from C++ STL container and add my own methods to it. The rationale was such that to the clients, it will look act a regular list, yet has application-specific methods they can readily be called.
这很好用,但我已经阅读了很多关于不继承 STL 的帖子.有人可以就我如何以更好的方式编写下面的代码提供具体建议吗?
This works fine, but I have read numerous posts about not inheriting from STL. Can someone provide a concrete advice of how I might write the code below in a better way?
class Item
{
int a;
int b;
int c;
int SpecialB()
{
return a * b + c;
}
};
class ItemList : public std::vector<Item>
{
int MaxA()
{
if( this->empty() )
throw;
int maxA = (*this)[0].a;
for( int idx = 1; idx < this->size(); idx++ )
{
if( (*this)[idx].a > maxA )
{
maxA = (*this)[idx].a;
}
}
return maxA;
}
int SpecialB()
{
if( this->empty() )
throw;
int specialB = (*this)[0].SpecialB();
for( int idx = 1; idx < this->size(); idx++ )
{
if( (*this)[idx].SpecialB() < specialB )
{
specialB -= (*this)[idx].c;
}
}
return specialB;
}
int AvgC()
{
if( this->empty() )
throw;
int cSum = 0;
for( int idx = 0; idx < this->size(); idx++ )
{
cSum += (*this)[idx].c;
}
return cSum / this->size(); // average
}
};
编辑:感谢您提供一系列深思熟虑的答案.我将改为创建辅助函数,从现在开始不再继承 STL 容器.
EDIT: Thanks for a bunch of thoughtful answers. I will create helper functions instead and from now on will never inherit from STL containers.
推荐答案
为什么需要以这种方式扩展向量?
why you need extend vector in this way?
对您的函子使用标准 .
例如
use standard <algorithm>
with your functors.
e.g.
std::min_element
, std::max_element
int max_a = std::max_element
(
v.begin(),
v.end(),
boost::bind(
std::less< int >(),
bind( &Item::a, _1 ),
bind( &Item::a, _2 )
)
)->a;
std::accumulate
- 对于计算平均值
std::accumulate
- for calculate avarage
const double avg_c = std::accumulate( v.begin(), v.end(), double( 0 ), boost::bind( Item::c, _1 ) ) / v.size(); // ofcourse check size before divide
您的 ItemList::SpecialB() 可以重写为:
your ItemList::SpecialB() could be rewrited as:
int accumulate_func( int start_from, int result, const Item& item )
{
if ( item.SpecialB() < start_from )
{
result -= item.SpecialB();
}
return result;
}
if ( v.empty() )
{
throw sometghing( "empty vector" );
}
const int result = std::accumulate( v.begin(), v.end(), v.front(), boost::bind( &accumulate_func, v.front(), _1, _2 ) );
顺便说一句:如果您不需要访问成员,则不需要继承.
BTW: if you don't need access to members, you don't need inheritance.
这篇关于关于使用用户定义方法扩展 C++ STL 容器的更好方法的建议的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:关于使用用户定义方法扩展 C++ STL 容器的更好方法的建议
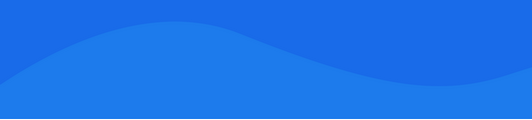
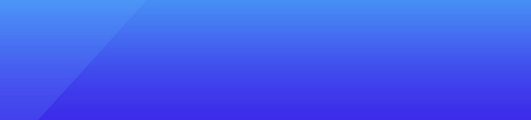
基础教程推荐
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 使用从字符串中提取的参数调用函数 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01