Catching exceptions from a constructor means that my instance is out of scope afterward(从构造函数捕获异常意味着我的实例之后超出范围)
问题描述
我有一个类,它的构造函数可能会抛出异常.下面是一些可以捕获异常的代码:
I have a class whose constructor may throw an exception. Here’s some code that will catch the exception:
try {
MyClass instance(3, 4, 5);
}
catch (MyClassException& ex) {
cerr << "There was an error creating the MyClass." << endl;
return 1;
}
但是当然在 try/catch 之后没有代码可以看到 instance
因为它现在超出了范围.解决此问题的一种方法是分别声明和定义 instance
:
But of course no code after the try/catch can see instance
because it’s now out of scope. One way to resolve this would be to declare and define instance
separately:
MyClass instance;
try {
MyClass instance(3, 4, 5);
}
...
除了我的类没有合适的零参数构造函数.事实上,这里的这种情况是唯一一个这样的构造函数甚至有意义的情况:MyClass
对象旨在是不可变的,从某种意义上说,它的数据成员在构造后都不会改变.如果我要添加一个零参数构造函数,我需要引入一些实例变量,例如 is_initialized_
然后检查每个方法以确保该变量之前是 true
进行.对于这样一个简单的模式来说,这似乎太过冗长了.
except that my class doesn’t have the appropriate zero-argument constructor. In fact, this case right here is the only one in which such a constructor would even make sense: the MyClass
object is intended to be immutable, in the sense that none of its data members change after construction. If I were to add a zero-argument constructor I’d need to introduce some instance variable like is_initialized_
and then have every method check to make sure that that variable is true
before proceeding. That seems like far too much verbosity for such a simple pattern.
处理这种事情的惯用方式是什么?我是否需要接受它并允许在初始化之前声明我的类的实例?
What is the idiomatic way to deal with this kind of thing? Do I need to suck it up and allow instances of my class to be declared before they’re initialized?
推荐答案
您应该在 try
块中内做您需要做的一切:
You should be doing everything you need to do inside the try
block:
try {
MyClass instance(3, 4, 5);
// Use instance here
}
catch (MyClassException& ex) {
cerr << "There was an error creating the MyClass." << endl;
return 1;
}
毕竟只有在try
块内,instance
才被成功创建,可以使用.
After all, it is only within the try
block that instance
has been successfully created and so can be used.
我确实想知道您的 catch
块是否真的在处理异常.如果你不能做任何事情来解决这种情况,你应该让它传播.
I do wonder whether your catch
block is really handling the exception. If you can't do anything to resolve the situation, you should be letting it propagate.
这篇关于从构造函数捕获异常意味着我的实例之后超出范围的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从构造函数捕获异常意味着我的实例之后超出范围
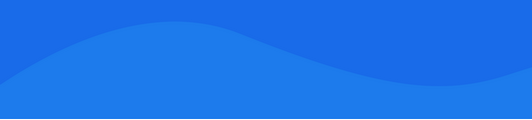
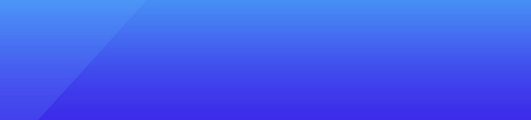
基础教程推荐
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 使用从字符串中提取的参数调用函数 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01