Linked List, insert at the end C++(链表,在末尾插入 C++)
问题描述
我正在编写一个简单的函数来插入到 C++ 链表的末尾,但最后它只显示了第一个数据.我无法弄清楚出了什么问题.这是功能:
I was writing a simple function to insert at the end of a linked list on C++, but finally it only shows the first data. I can't figure what's wrong. This is the function:
void InsertAtEnd (node* &firstNode, string name){
node* temp=firstNode;
while(temp!=NULL) temp=temp->next;
temp = new node;
temp->data=name;
temp->next=NULL;
if(firstNode==NULL) firstNode=temp;
}
推荐答案
你写的是:
如果
firstNode
为空,它被替换为单个节点temp
没有下一个节点(没有人的next
是temp
)
if
firstNode
is null, it's replaced with the single nodetemp
which has no next node (and nobody'snext
istemp
)
否则,如果 firstNode
不为 null,则什么都不会发生,除了 temp
节点被分配和泄漏.
Else, if firstNode
is not null, nothing happens, except that the temp
node is allocated and leaked.
下面是更正确的代码:
void insertAtEnd(node* &first, string name) {
// create node
node* temp = new node;
temp->data = name;
temp->next = NULL;
if(!first) { // empty list becomes the new node
first = temp;
return;
} else { // find last and link the new node
node* last = first;
while(last->next) last=last->next;
last->next = temp;
}
}
另外,我建议向 node
添加一个构造函数:
Also, I would suggest adding a constructor to node
:
struct node {
std::string data;
node* next;
node(const std::string & val, node* n = 0) : data(val), next(n) {}
node(node* n = 0) : next(n) {}
};
它使您能够像这样创建 temp
节点:
Which enables you to create the temp
node like this:
node* temp = new node(name);
这篇关于链表,在末尾插入 C++的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:链表,在末尾插入 C++
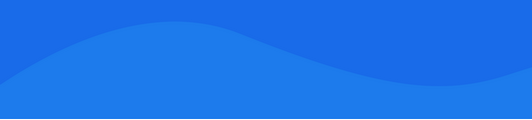
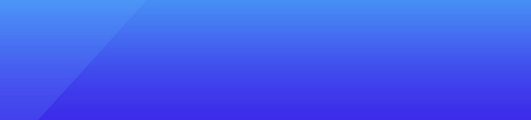
基础教程推荐
- 从 std::cin 读取密码 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01