Search Function in Linked List - C++(链表中的搜索函数 - C++)
问题描述
我的目标是生成一个函数来搜索列表中已有的数字并打印它已找到.
我最初的想法是按照我的删除功能搜索列表,直到找到一个数字(然后删除).
这似乎是对搜索功能进行编码的合乎逻辑的方式.如果这不正确,我将如何修改它以搜索我的列表并显示已找到一个号码?
我有节点 *head、*current 和 *temp 以及节点指针 next 和 number 作为 .h 文件上类中的数据类型.
谢谢.
注意 - 我在 search() 函数下使用了我的 remove() 函数.
My aim is to produce a function that searches for a number already in the list and print that it has been found.
My initial idea was to follow my remove function which searches through the list until it finds a number (to then delete).
This seemed the logical way to code the search function. If this isn't correct how would I modify it to search through my list and display that a number has been found?
I have nodes *head, *current and *temp as well as node pointer next and number as the data type in a class on a .h file.
Thank you.
NOTE - I used my remove() function under the search() function.
#include <iostream>
#include <string>
#include <fstream>
#include "LinkedList.h"
using namespace SDI;
int main()
{
LinkedList menu;
menu.insert(5);
menu.insert(4);
menu.insert(2);
menu.insert(3);
menu.insert(8);
menu.remove(4);
menu.reverse();
menu.display();
menu.search(2);
system("pause");
};
LinkedList::LinkedList()
{
head = NULL;
current = NULL;
temp = NULL;
};
LinkedList::~LinkedList()
{
};
void LinkedList::insert(int add) //insert function, data is stored in add from function body
{
Node* newnode = new Node; //definition of add node, make new node and make node* point to it
newnode->next = NULL; //point and set up to last node in the list (nothing)
newnode->number = add; //adds data to list
if (head != NULL) //if head is pointing to object then we have list
{
current = head; //make current pointer point to head
while (current->next != NULL) //check to see if end at list, is it the last node?
{
current = current->next; //advances current pointer to end of list
}
current->next = newnode; //adds new node next to value already stored
}
else
{
head = newnode; //if we don't have element in list
}
};
void LinkedList::remove(int remove) //remove function, data is stored in remove from function body
{
Node* remove1 = NULL; //searches through for same value in remove and deletes
temp = head;
current = head;
while (current != NULL && current->number != remove) //check if current node is one we want to delete...if not advance current pointer to next one
{
temp = current; //keep temp pointer one step behind
current = current->next; //advance to next node, traverse list till at the end
}
if (current == NULL) //pass through whole list and value not found
{
std::cout << "N/A
";
delete remove1; //removes spare number floating around in memory
}
else
{
remove1 = current; //pointing to value we want to delete
current = current->next; //advances current pointer to next node
temp->next = current; //stops hole that occurs in list, patches this up
if (remove1 == head) //if pointer is pointing to front of list
{
head = head->next; //advance the head to next
temp = NULL;
}
delete remove1;
}
};
void LinkedList::search(int searchNum)
{
Node* searchnumber = nullptr;
temp = head;
current = head;
while (current != NULL && current->number != searchNum)
{
temp = current;
current = current->next;
}
if (current != NULL)
{
searchnumber = current;
current = current->next;
std::cout << "-" << searchnumber << " Found";
}
else
{
std::cout << "N/A";
}
};
void LinkedList::display()
{
current = head; //point to start of list
while (current != NULL) //while it points to something in list
{
std::cout << current->number; //display list starting from start
current = current->next; //advance to next pointer
}
};
void LinkedList::reverse()
{
Node *new_head = nullptr; //create new head as we want it to start from last element
for (current = head; current;) //same as display, ask it to go through list from head then outside loop assign to new head and switch sides
{
temp = current; //keep temp pointer one step behind
current = current->next; //goes through each element in the list
temp->next = new_head; //scrolls through backwards from new head
new_head = temp;
}
head = new_head; //assign head to new head
};
推荐答案
您的搜索算法似乎有误.将其更改为:
Your search algorithm seems to be wrong. Change it to :
if (current != NULL) // (current == NULL) is wrong because it means the value wasn't found
{
searchnumber = current;
current = current->next;
std::cout << "-" << searchnumber->number << " Found"; // here searchnumber is the node's address. You need to print its value, so use searchnumber->number
}
并且您不需要删除节点,直到找到所需的值.
您可以仅使用搜索算法来查找列表中是否已有数字.如果这就是你想要的.
And you don't need to remove nodes till you find the desired value.
You can just use your search algorithm to find if a number already in the list. If that's what you want.
这篇关于链表中的搜索函数 - C++的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:链表中的搜索函数 - C++
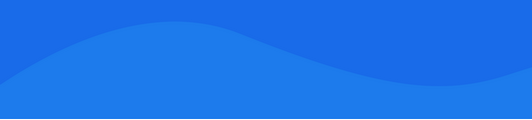
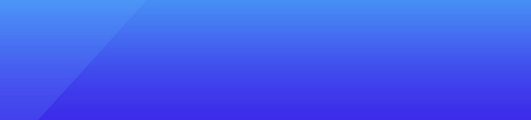
基础教程推荐
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- Windows Media Foundation 录制音频 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01