Reference variable with error, must be initialized in constructor base/member initializer(有错误的引用变量,必须在构造函数基类/成员初始化器中初始化)
问题描述
当我尝试编译下面的源代码时出现以下错误.任何人都可以描述为什么存在此错误以及我如何解决它?
I got following error when I try to compile the source-code below. Can anybody describe why this error exists and how I can fix it?
错误 1 错误 C2758:'A::s_':必须在构造函数基类/成员初始化器中初始化
#include <iostream>
#include <string>
using namespace std;
class A
{
public:
A(string& s) : s_(s) { cout << "A::ctor" << endl; }
A(const A& rhs) { cout << "A::copy" << endl; }
~A() { cout << "A::dtor" << endl; }
A& operator=(const A& rhs) { cout << "A::copyassign" << endl; }
private:
string& s_;
};
int main()
{
return 0;
}
推荐答案
首先,你的 A::s_
是对 std::string
的引用;这意味着它正在引用某处必须存在的东西.
First of all, your A::s_
is a reference to a std::string
; that means that it's referencing something that must exists somewhere.
由于他的引用类型,以及引用必须在创建时初始化的事实,您必须在所有 A
中初始化 A::s_
> 构造函数(由其他用户指出):
Due of his reference type, and the fact that the references must be initialized at the moment they're created, you must initialize A::s_
in ALL the A
constructors (as pointed by other users):
class A
{
public:
A(string& s) : s_(s)
{ cout << "A::ctor" << endl; }
A(const A& rhs) : s_(rhs.s_) // <-- here too!!
{ cout << "A::copy" << endl; }
~A()
{ cout << "A::dtor" << endl; }
A& operator=(const A& rhs)
{ cout << "A::copyassign" << endl; }
private:
string& s_;
};
现在,回到我提到的第一件事;A::s_
必须引用存在的东西,所以一定要注意一些东西,看看下面的代码:
And now, back to the first thing I mentioned; the A::s_
must reference something that exists, so you must be aware of some things, take a look at the following code:
int main()
{
// New A instance:
A a("hello world");
return 0;
}
构造这个 A
实例,我们提供了一个 const char[12]
值,这个值是一个临时的 std::string
创建并提供给 A::A(string& s)
构造函数.构造函数结束后 A::s_
在哪里引用?创建的临时 std::string
会发生什么?当 A
构造函数结束时,它的生命周期会延长还是死亡?您确定需要参考吗?
Constructing this A
instance we're providing a const char[12]
value, with this value a temporary std::string
is created and is given to the A::A(string& s)
constructor. Where A::s_
is referencing after the constructor ends? What happens with the temporary std::string
created? It's lifetime is extended or it just die when the A
constructor ends? Are you sure that a reference is what you need?
std::string s("hello world");
int main()
{
// New A instance:
A a(s);
return 0;
}
使用上面的代码,创建了一个新的 A
实例,调用相同的 A::A(string& s)
构造函数,但提供的字符串位于全局作用域,因此它不会被销毁,并且 a
实例中的 A::s_
将在其整个生命周期中引用一个有效的字符串,但真正的威胁在于复制构造函数:
With the code above, a new A
instance is created calling the same A::A(string& s)
constructor, but with a provided string lying in the global scope, so it doesn't be destroyed and the A::s_
from the a
instance would reference a valid string all its lifetime, but the real threat is in the copy constructor:
std::string s("hello world");
int main()
{
A a(s); // a.s_ references the global s.
A b(a); // b.s_ references the a.s_ that references the global s.
return 0;
}
复制的对象值将引用给定对象的std::string
!这就是你想要的吗?
The copied object value will reference the std::string
of the given object! Is that what you want?
这篇关于有错误的引用变量,必须在构造函数基类/成员初始化器中初始化的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:有错误的引用变量,必须在构造函数基类/成员初始化器中初始化
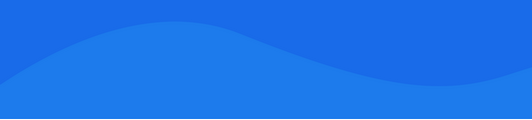
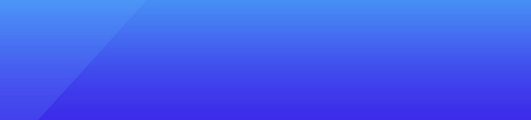
基础教程推荐
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 从 std::cin 读取密码 2021-01-01