Why is a default constructor required when storing in a map?(为什么在映射中存储时需要默认构造函数?)
问题描述
我收到错误:
error: no matching function for call to 'A::A()'
note: candidates are: A::A(const A&)
note: A::A(const std::string&, size_t)
从这里:
#include <map>
#include <string>
using std::map;
using std::string;
class A {
public:
string path;
size_t size;
A (const string& p, size_t s) : path(p), size(s) { }
A (const A& f) : path(f.path), size(f.size) { }
A& operator=(const A& rhs) {
path = rhs.path;
size = rhs.size;
return *this;
}
};
int main(int argc, char **argv)
{
map<string, A> mymap;
A a("world", 1);
mymap["hello"] = a; // <----- here
A b(mymap["hello"]); // <----- and here
}
请告诉我为什么代码需要一个没有参数的构造函数.
Please tell me why the code wants a constructor with no parameters.
推荐答案
mymap["hello"]
可以尝试创建一个值初始化的A
,所以默认构造函数是必需的.
mymap["hello"]
can attempt to create a value-initialized A
, so a default constructor is required.
如果您使用类型 T
作为 map
值(并计划通过 operator[]
访问值),则需要默认构造 - 即您需要一个无参数(默认)构造函数.operator[]
如果没有找到提供键的值,映射上的 operator[]
将对映射值进行值初始化.
If you're using a type T
as a map
value (and plan to access value via operator[]
), it needs to be default-constructible - i.e. you need a parameter-less (default) constructor. operator[]
on a map will value-initialize the mapped value if a value with the key provided is not found.
这篇关于为什么在映射中存储时需要默认构造函数?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:为什么在映射中存储时需要默认构造函数?
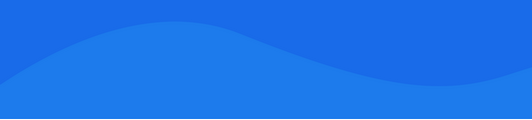
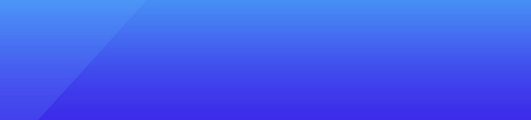
基础教程推荐
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01