When do we need a private constructor in C++?(我们什么时候需要 C++ 中的私有构造函数?)
问题描述
我有一个关于 C++ 中私有构造函数的问题.如果构造函数是私有的,我如何创建类的实例?
I have a question about private constructors in C++. If the constructor is private, how can I create an instance of the class?
我们应该在类内部有一个 getInstance() 方法吗?
Should we have a getInstance() method inside the class?
推荐答案
private
构造函数有几种情况:
There are a few scenarios for having private
constructors:
限制除
friend
以外的所有对象的创建;在这种情况下,所有构造函数都必须是private
Restricting object creation for all but
friend
s; in this case all constructors have to beprivate
class A
{
private:
A () {}
public:
// other accessible methods
friend class B;
};
class B
{
public:
A* Create_A () { return new A; } // creation rights only with `B`
};
限制特定类型的构造函数(即复制构造函数、默认构造函数).例如std::fstream
不允许通过这种不可访问的构造函数进行复制
Restricting certain type of constructor (i.e. copy constructor, default constructor). e.g. std::fstream
doesn't allow copying by such inaccessible constructor
class A
{
public:
A();
A(int);
private:
A(const A&); // C++03: Even `friend`s can't use this
A(const A&) = delete; // C++11: making `private` doesn't matter
};
要有一个公用的委托构造函数,不应该暴露给外部世界:
To have a common delegate constructor, which is not supposed to be exposed to the outer world:
class A
{
private:
int x_;
A (const int x) : x_(x) {} // common delegate; but within limits of `A`
public:
A (const B& b) : A(b.x_) {}
A (const C& c) : A(c.foo()) {}
};
对于 单例模式,当单例 class
不可继承时(如果是可继承然后使用 protected
构造函数)
For singleton patterns when the singleton class
is not inheritible (if it's inheritible then use a protected
constructor)
class Singleton
{
public:
static Singleton& getInstance() {
Singleton object; // lazy initialization or use `new` & null-check
return object;
}
private:
Singleton() {} // make `protected` for further inheritance
Singleton(const Singleton&); // inaccessible
Singleton& operator=(const Singleton&); // inaccessible
};
这篇关于我们什么时候需要 C++ 中的私有构造函数?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:我们什么时候需要 C++ 中的私有构造函数?
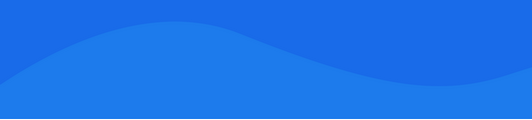
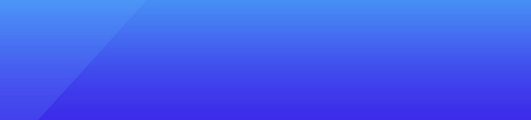
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01