Is it possible to iterate an mpl::vector at run time without instantiating the types in the vector?(是否可以在运行时迭代 mpl::vector 而无需实例化向量中的类型?)
问题描述
通常,我会使用 boost::mpl::for_each<>()
来遍历 boost::mpl::vector
,但这需要一个函子模板函数声明如下:
Generally, I would use boost::mpl::for_each<>()
to traverse a boost::mpl::vector
, but this requires a functor with a template function declared like the following:
template
我的问题是我不希望对象 T 被 for_each<>
实例化.我根本不需要 operator()
中的 T 参数.有没有办法实现这一点,或者 for_each<>
的替代方法,它不会将 T 类型的对象传递给模板函数?
My problem with this is that I don't want the object T to be instantiated by for_each<>
. I don't need the T parameter in the operator()
at all. Is there a way to accomplish this, or an alternative to for_each<>
that doesn't pass an object of type T to the template function?
最理想的是,我希望 operator() 定义如下所示:
Optimally, I would like the operator() definition to look like this:
template
当然,我不希望在调用之前对 T 进行实例化.也欢迎任何其他提示/建议.
And of course, I don't want T to be instantiated at all prior to the call. Any other tips/suggestions are also welcome.
推荐答案
有趣的问题!据我所知,Boost.MPL 似乎没有提供这样的算法.但是,使用迭代器编写自己的代码应该不会太困难.
Interesting question! As far as I can tell, Boost.MPL does not seem to provide such an algorithm. However, writing your own should not be too difficult using iterators.
这是一个可能的解决方案:
Here is a possible solution:
#include <boost/mpl/begin_end.hpp>
#include <boost/mpl/next_prior.hpp>
#include <boost/mpl/vector.hpp>
using namespace boost::mpl;
namespace detail {
template < typename Begin, typename End, typename F >
struct static_for_each
{
static void call( )
{
typedef typename Begin::type currentType;
F::template call< currentType >();
static_for_each< typename next< Begin >::type, End, F >::call();
}
};
template < typename End, typename F >
struct static_for_each< End, End, F >
{
static void call( )
{
}
};
} // namespace detail
template < typename Sequence, typename F >
void static_for_each( )
{
typedef typename begin< Sequence >::type begin;
typedef typename end< Sequence >::type end;
detail::static_for_each< begin, end, F >::call();
}
[命名可能不是很好,但很好...]
[The naming may not be very well chosen, but well...]
以下是您将如何使用此算法:
Here is how you would use this algorithm:
struct Foo
{
static void staticMemberFunction( )
{
std::cout << "Foo";
}
};
struct Bar
{
static void staticMemberFunction( )
{
std::cout << "Bar";
}
};
struct CallStaticMemberFunction
{
template < typename T >
static void call()
{
T::staticMemberFunction();
}
};
int main()
{
typedef vector< Foo, Bar > sequence;
static_for_each< sequence, CallStaticMemberFunction >(); // prints "FooBar"
}
这篇关于是否可以在运行时迭代 mpl::vector 而无需实例化向量中的类型?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:是否可以在运行时迭代 mpl::vector 而无需实例化向量中的类型?
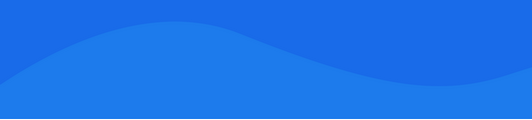
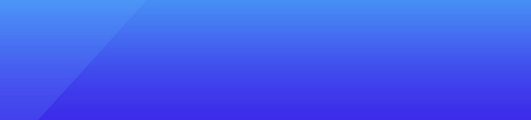
基础教程推荐
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- Windows Media Foundation 录制音频 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01