Get index in C++11 foreach loop(在 C++11 foreach 循环中获取索引)
问题描述
在 C++11 foreach 循环中是否有一种方便的方法来获取当前容器条目的索引,例如 python 中的 enumerate
:
Is there a convenient way to get the index of the current container entry in a C++11 foreach loop, like enumerate
in python:
for idx, obj in enumerate(container):
pass
我可以想象一个迭代器也可以返回索引或类似的东西.
I could imagine an iterator that can also return the index or similar.
当然我可以有一个计数器,但迭代器通常不保证它们在容器上迭代的顺序.
Of course I could have a counter, but often iterators don't give guarantees of the order they iterate over a container.
推荐答案
可以在此处找到您请求的功能的良好实现:
A good implementation of the feature you are requested can be found here:
https://github.com/ignatz/pythonic
背后的想法是,您使用自定义迭代器构建一个包装结构来进行计数.下面是一个非常小的示例性实现来说明这个想法:
The idea behind is, that you build a wrapper struct with a custom iterator that does the counting. Below is a very minimal exemplary implementation to illustrate the idea:
// Distributed under the terms of the GPLv2 or newer
#include <iostream>
#include <vector>
#include <tuple>
// Wrapper class
template <typename T>
class enumerate_impl
{
public:
// The return value of the operator* of the iterator, this
// is what you will get inside of the for loop
struct item
{
size_t index;
typename T::value_type & item;
};
typedef item value_type;
// Custom iterator with minimal interface
struct iterator
{
iterator(typename T::iterator _it, size_t counter=0) :
it(_it), counter(counter)
{}
iterator operator++()
{
return iterator(++it, ++counter);
}
bool operator!=(iterator other)
{
return it != other.it;
}
typename T::iterator::value_type item()
{
return *it;
}
value_type operator*()
{
return value_type{counter, *it};
}
size_t index()
{
return counter;
}
private:
typename T::iterator it;
size_t counter;
};
enumerate_impl(T & t) : container(t) {}
iterator begin()
{
return iterator(container.begin());
}
iterator end()
{
return iterator(container.end());
}
private:
T & container;
};
// A templated free function allows you to create the wrapper class
// conveniently
template <typename T>
enumerate_impl<T> enumerate(T & t)
{
return enumerate_impl<T>(t);
}
int main()
{
std::vector<int> data = {523, 1, 3};
for (auto x : enumerate(data))
{
std::cout << x.index << ": " << x.item << std::endl;
}
}
这篇关于在 C++11 foreach 循环中获取索引的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 C++11 foreach 循环中获取索引
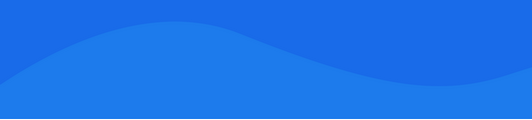
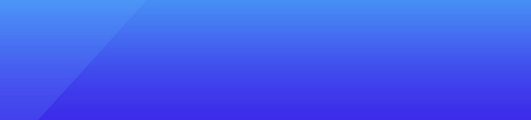
基础教程推荐
- Windows Media Foundation 录制音频 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01