boost::variant - why is quot;const char*quot; converted to quot;boolquot;?(boost::variant - 为什么是“const char*;转换为“布尔?)
问题描述
我已经声明了一个 boost::variant
,它接受三种类型:string
、bool
和 int
.以下代码显示我的变体接受 const char*
并将其转换为 bool
.boost::variant
接受和转换不在其列表中的类型是正常行为吗?
I have declared a boost::variant
which accepts three types: string
, bool
and int
. The following code is showing that my variant accepts const char*
and converts it to bool
. Is it a normal behavior for boost::variant
to accept and convert types not on its list?
#include <iostream>
#include "boost/variant/variant.hpp"
#include "boost/variant/apply_visitor.hpp"
using namespace std;
using namespace boost;
typedef variant<string, bool, int> MyVariant;
class TestVariant
: public boost::static_visitor<>
{
public:
void operator()(string &v) const
{
cout << "type: string -> " << v << endl;
}
template<typename U>
void operator()(U &v)const
{
cout << "type: other -> " << v << endl;
}
};
int main(int argc, char **argv)
{
MyVariant s1 = "some string";
apply_visitor(TestVariant(), s1);
MyVariant s2 = string("some string");
apply_visitor(TestVariant(), s2);
return 0;
}
输出:
类型:其他 -> 1
类型:字符串 -> 一些字符串
type: other -> 1
type: string -> some string
如果我从 MyVariant 中删除 bool 类型并将其更改为:
If I remove the bool type from MyVariant and change it to this:
typedef variant<string, int> MyVariant;
const char*
不再转换为 bool
.这次它被转换为 string
,这是新的输出:
const char*
is no more converted to bool
. This time it's converted to string
and this is the new output:
类型:字符串 -> 一些字符串
类型:字符串 -> 一些字符串
type: string -> some string
type: string -> some string
这表明variant
尝试先将其他类型转换为bool
,然后再转换为string
.如果类型转换是不可避免的并且应该总是发生,有什么方法可以将转换为 string
赋予更高的优先级?
This indicates that variant
tries to convert other types first to bool
and then to string
. If the type conversion is something inevitable and should always happen, is there any way to give conversion to string
a higher priority?
推荐答案
我不认为这与 boost::variant
有什么特别关系,而是关于重载解析选择了哪个构造函数.重载函数也会发生同样的事情:
I don't think this is anything particularly to do with boost::variant
, it's about which constructor gets selected by overload resolution. The same thing happens with an overloaded function:
#include <iostream>
#include <string>
void foo(bool) {
std::cout << "bool
";
}
void foo(std::string) {
std::cout << "string
";
}
int main() {
foo("hi");
}
输出:
bool
我不知道有什么方法可以更改 Variant 的构造函数
I don't know of a way to change what constructors a Variant has [edit: as James says, you can write another class that uses the Variant in its implementation. Then you can provide a const char*
constructor that does the right thing.]
也许您可以更改 Variant 中的类型.另一个重载示例:
Maybe you could change the types in the Variant. Another overloading example:
struct MyBool {
bool val;
explicit MyBool(bool val) : val(val) {}
};
void bar(MyBool) {
std::cout << "bool
";
}
void bar(const std::string &) {
std::cout << "string
";
}
int main() {
bar("hi");
}
输出:
string
不幸的是,现在您必须编写 bar(MyBool(true))
而不是 foo(true)
.更糟糕的是,对于带有 string/bool/int
的变体,如果您只是将其更改为 string/MyBool/int
的变体,则 MyVariant(true)
将调用 int
构造函数.
Unfortunately now you have to write bar(MyBool(true))
instead of foo(true)
. Even worse in the case of your variant with string/bool/int
, if you just change it to a variant of string/MyBool/int
then MyVariant(true)
would call the int
constructor.
这篇关于boost::variant - 为什么是“const char*";转换为“布尔"?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:boost::variant - 为什么是“const char*";转换为“布尔"?
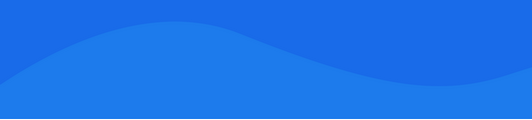
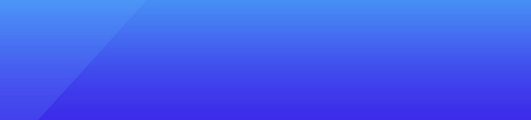
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01