making non-shared copies of boost::interprocess shared memory objects(制作 boost::interprocess 共享内存对象的非共享副本)
问题描述
我已经实现了各种旨在用于boost::interprocess
共享内存段的类.他们所有的构造函数都使用 allocator<void,segment_manager>
引用——有些在我写的定义中是明确的(比如下面的 Foo
构造函数),有些只是因为这就是 boost 容器定义要求,在 boost 库代码中,我不应该更改(如下面的 IndexVector
).
I have implemented various classes that are designed to be used in boost::interprocess
shared memory segments. All their constructors employ allocator<void,segment_manager>
references—some explicitly in the definitions I have written (like the Foo
constructor below) and some simply because that's what the boost container definition requires, in boost library code that I should not be changing (like the IndexVector
below).
#include <boost/interprocess/managed_shared_memory.hpp>
#include <boost/interprocess/allocators/allocator.hpp>
#include <boost/interprocess/containers/vector.hpp>
typedef boost::interprocess::managed_shared_memory Segment;
typedef boost::interprocess::managed_shared_memory::segment_manager SegmentManager;
typedef boost::interprocess::allocator< void, SegmentManager > Allocator;
typedef size_t Index;
typedef boost::interprocess::allocator< Index, SegmentManager > IndexAllocator;
typedef boost::interprocess::vector< Index, IndexAllocator > IndexVector;
class Foo
{
public:
Foo( const Allocator & alloc ) : mData( alloc ) {}
~Foo() {}
private:
IndexVector mData;
};
大多数情况下,这些对象位于共享内存中.但有时我想在非共享内存中创建它们的副本.我的问题是:我是否必须定义一个完全不同的类(例如 Foo_Nonshared
),其中包含不同的成员类型(std::vector
而不是我的共享 >IndexVector
类型)并在它们之间提供复制/转换功能?这将是大量的工作和大量愚蠢的重复.我可以通过为现有的 Foo
类提供替代构造函数来减少重复,但是我不知道如何在没有分配器的情况下初始化 IndexVector
成员.
Mostly, these objects sit in shared memory. But I sometimes want to create copies of them in non-shared memory. My question is this: do I have to define a whole different class (e.g. Foo_Nonshared
) containing different member types (std::vector<Index>
instead of my shared IndexVector
type) and provide copy/conversion functions between them? That will be a lot of work and a lot of stupid duplication. I could reduce duplication by providing an alternative constructor to the existing Foo
class, but then I wouldn't know how to initialize the IndexVector
member without an allocator.
或者有什么好的捷径吗?我正在想象某种特定的 allocator
实例,我可以将它传递给 Foo()
,因此它将传递给 IndexVector
构造函数,这将被两者识别为在非共享内存中分配".这样的事情存在吗?是否有用于管理普通非共享内存的虚拟段管理器"?或者有其他方法可以解决这个问题吗?
Or is there some nice shortcut? I'm imagining some sort of particular allocator
instance that I can pass to Foo()
, and which will hence be passed on to the IndexVector
constructor, which will be recognized by both as meaning "allocate in non-shared memory". Does such a thing exist? Is there a "dummy segment manager" for managing vanilla non-shared memory? Or are there other ways around this problem?
我希望得到与 C++03 兼容的答案,尽管我也有兴趣学习 C++11+ 的做事方式.
I'm hoping for C++03-compatible answers even though I'm also interested to learn the C++11+ ways of doing things.
更新以下问题被标记为重复:我已阅读这些以前的类似问题:
Update following question being marked as duplicate: I have read these previous similar questions:
- boost::interprocess 容器的容器不在共享内存中一个>
- boost::interprocess 容器的容器不在共享内存中复制
并试图概括我在那里看到的内容,有一些成功和一些失败(见下面的列表).有一些编译器错误我无法解决,标记为 ERROR — 特别是我无法弄清楚如何实例化迭代这些高度元"容器的成员的方法.但是无论有没有这些错误,我还无法看到如何将模板的模板变成可维护的解决方案(实际上,我的对象包含其他复杂对象的容器,其中包含更多容器,AFAICS 使语法复杂化,超出理智...见标有嗯"的部分).
and have tried to generalize what I see there, with some successes and some failures (see listing below). There are a few compiler errors that I haven't been able to resolve, marked ERROR—in particular I cannot figure out how to instantiate methods that iterate over the members of these highly "meta" containers. But with or without those errors, I cannot yet see how to make templates-of-templates into a maintainable solution (my objects, in reality, contain containers of other complex objects, which contain further containers, which AFAICS complicates the syntax beyond sanity... see the part marked "hmm").
我想,最后,我可能不得不重新设计以避免在共享内存和堆内存中使用相同的对象.
I guess, in the end, I might have to re-design to avoid having the same objects in shared and heap memory.
#include <boost/interprocess/managed_shared_memory.hpp>
#include <boost/interprocess/allocators/allocator.hpp>
#include <boost/interprocess/containers/vector.hpp>
namespace bip = boost::interprocess; // warning: C++11 alias declaration
template <typename T, template<typename...> class Allocator> // warning: C++11 variadic template
using Vector = bip::vector< T, Allocator<T>>; // warning: C++11 alias declaration
// this seems to work to get some of the nested <>ness under control.
// But I can't figure out how to create an iterator to this kind of type (see errors below)
// what once were classes are now class templates
template <template<typename...> class Allocator> // warning: C++11 variadic template
class Bar
{
public:
Bar( const Allocator<void> & alloc ) : mInts( alloc ) {}
~Bar() {}
void Report( void );
private:
Vector< int, Allocator > mInts;
};
template <template<typename...> class Allocator> // warning: C++11 variadic template
class Foo
{
public:
Foo( const Allocator<void> & alloc ) : mBars( alloc ) {}
~Foo() {}
void Report( void );
private:
Vector< Bar<Allocator>, Allocator > mBars; // hmm, with more complex structures this is going
// to get unmanageably< nested< very< quickly > > > ...
};
// Define allocator templates
template <typename T>
using HeapAllocator = std::allocator<T>; // warning: C++11 alias declaration
template <typename T>
using ShmemAllocator = bip::allocator<T, bip::managed_shared_memory::segment_manager>; // warning: C++11 alias declaration
// Define two class variants: one for use on the heap and one for use in shared memory
using HeapFoo = Foo< HeapAllocator >; // warning: C++11 alias declaration
using ShmemFoo = Foo< ShmemAllocator >; // warning: C++11 alias declaration
// Try to define methods (unsuccessful so far because of the iterators,
// but they compile OK if the function bodies are left empty):
template <template<typename...> class Allocator> // warning: C++11 variadic template
void
Bar< Allocator >::Report( void )
{
std::cout << "[";
Vector< int, Allocator >::iterator it;
// ERROR: ^~~~~ expected ';' after expression
for( it = mInts.begin(); it += mInts.end(); it++ )
std::cout << ( it == mInts.begin() ? "" : ", " ) << *it;
std::cout << "]
";
}
template <template<typename...> class Allocator> // warning: C++11 variadic template
void
Foo< Allocator >::Report( void )
{
Vector< Bar< Allocator >, Allocator >::iterator it;
// ERROR: ^~~~~ expected ';' after expression
for( it = mBars.begin(); it += mBars.end(); it++ )
it->Report();
std::cout << "
";
}
int main( void )
{
struct shm_remove
{
shm_remove() { bip::shared_memory_object::remove( "MySharedMemory" ); }
~shm_remove() { bip::shared_memory_object::remove( "MySharedMemory" ); }
} remover;
bip::managed_shared_memory seg( bip::create_only, "MySharedMemory", 65536 );
ShmemAllocator< void > shalloc( seg.get_segment_manager() );
HeapAllocator< void > halloc;
HeapFoo foo1( halloc );
ShmemFoo foo2( shalloc );
foo1.Report();
foo2.Report();
}
推荐答案
好的,您已经遇到了Frequently Annoying Edgecase",即模板-模板参数不是 C++ 中的一等公民(您不能传递它们/typedef 它们):
Ok, you've run into the Frequently Annoying Edgecase that template-template arguments aren't first class citizens in C++ (you cannot pass them around/typedef them):
- 如何传输模板?
我们该怎么办?
allocator::rebind
分配器有一个重新绑定机制,我敢说正是因为这个.所以你可以传递一个 alloc
就好像它是开放模板一样,因为你总是可以通过执行 Alloc::rebind
.
Allocators have a rebind mechanism, I daresay precisely because of this. So you can pass a alloc<void>
as if it is the open template, because you can always get from there to a sibling allocator type by doing Alloc::rebind<T>::other
.
除此之外,分配器通常具有执行此重新绑定的转换构造函数,因此您无需在许多采用分配器的地方过于具体
Add to this the fact that allocators usually have conversion constructors that do this rebinding, you don't need to be overly specific in many places taking allocators
在 c++11 中,引入了 scoped_allocator
以避免必须在许多地方手动传递 allocator
实例,这些地方将进行内部构造元素(例如 emplace_back
).
in c++11, scoped_allocator
s have been introduced to avoid having to manually pass allocator
instances in a number of places that will do internal construction of elements (e.g. emplace_back
).
有一个库魔法,它会自动从容器的 scoped_allocator
添加分配器实例作为最后一个构造函数参数(默认情况下).Boost Container 库已将 scoped_allocator_adaptor
概念向后移植到 c++03,以便您可以使用它.
There's library magic in place that will automatically add the allocator instance from the container's scoped_allocator
as the last constructor argument (by default). Boost Container library has backported the scoped_allocator_adaptor
concept to c++03 so you can use it.
这是一个完整的示例,向您展示了如何解决您遇到的问题,以及如何将基于堆的 Bar
实例与共享内存 Foo
混合使用> 实例:
Here's a full sample that shows you how to solve the issues you had, and also, how you can mix the heap-based Bar
instances with the shared-memory Foo
instance:
foo2.add(bar1); // this works because of ... MAGIC!
由于上面提到的 scoped_allocator
而起作用.
Which works due to the scoped_allocator
mentioned above.
生活在 Coliru强>
#include <boost/interprocess/managed_shared_memory.hpp>
#include <boost/interprocess/allocators/allocator.hpp>
#include <boost/interprocess/containers/vector.hpp>
#include <boost/container/scoped_allocator.hpp>
namespace bip = boost::interprocess;
namespace generic {
template <typename T, typename Alloc/* = std::allocator<T>*/ >
using vector = bip::vector<T, typename Alloc::template rebind<T>::other >;
template <typename Alloc> struct Bar {
typedef Alloc allocator_type; // ties in with uses_allocator/scoped_allocator
// only require allocator if not default-constructible
Bar(Alloc alloc = Alloc()) : mInts(alloc) {}
// conversion constructor so we can convert between allocators
template <typename OtherAlloc>
Bar(Bar<OtherAlloc> const& rhs, Alloc alloc = Alloc())
: mInts(rhs.mInts.begin(), rhs.mInts.end(), alloc)
{
}
void Report() const;
void add(int i) { mInts.emplace_back(i); }
private:
template<typename OtherAlloc> friend struct Bar; // we can see each other's mInts
typedef vector<int, Alloc> ints_t;
ints_t mInts;
};
template <typename Alloc> struct Foo {
typedef Alloc allocator_type; // ties in with uses_allocator/scoped_allocator
Foo(Alloc alloc = Alloc()) : mBars(alloc) {}
void Report() const;
template <typename Bar>
void add(Bar const& bar) { mBars.emplace_back(bar); }
private:
typedef vector<Bar<Alloc>, Alloc> mbars_t;
mbars_t mBars;
};
}
namespace heap {
using VAlloc = std::allocator<void>;
using Bar = generic::Bar<VAlloc>;
using Foo = generic::Foo<VAlloc>;
}
namespace shared {
using VAlloc = boost::container::scoped_allocator_adaptor<bip::allocator<void, bip::managed_shared_memory::segment_manager> >;
using Bar = generic::Bar<VAlloc>;
using Foo = generic::Foo<VAlloc>;
}
template <typename Alloc> void generic::Bar<Alloc>::Report() const {
std::cout << "[";
for (typename ints_t::const_iterator it = mInts.begin(); it != mInts.end(); it++)
std::cout << (it == mInts.begin() ? "" : ", ") << *it;
std::cout << "]
";
}
template <typename Alloc>
void generic::Foo<Alloc>::Report() const {
for (typename mbars_t::const_iterator it = mBars.begin(); it != mBars.end(); it++)
it->Report();
std::cout << "
";
}
int main(void) {
struct shm_remove {
shm_remove() { bip::shared_memory_object::remove("MySharedMemory"); }
~shm_remove() { bip::shared_memory_object::remove("MySharedMemory"); }
} remover;
///////////////////////////////////
// heap based:
std::cout << "Heap based storage:
";
heap::Foo foo1;
heap::Bar bar1;
bar1.add(42);
bar1.add(2);
bar1.add(-99);
foo1.add(bar1);
foo1.Report();
/////////////////////////////////
std::cout << "Shared memory storage:
";
bip::managed_shared_memory seg(bip::create_only, "MySharedMemory", 65536);
shared::VAlloc shalloc(seg.get_segment_manager());
shared::Foo foo2(shalloc);
shared::Bar bar2(shalloc);
bar2.add(43);
bar2.add(3);
bar2.add(-98);
foo2.add(bar2); // of course this works
foo2.add(bar1); // this works because of ... MAGIC!
foo2.Report();
}
打印:
Heap based storage:
[42, 2, -99]
Shared memory storage:
[43, 3, -98]
[42, 2, -99]
这篇关于制作 boost::interprocess 共享内存对象的非共享副本的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:制作 boost::interprocess 共享内存对象的非共享副本
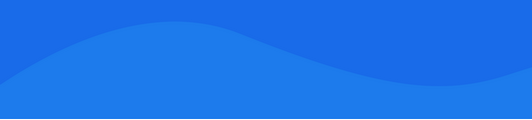
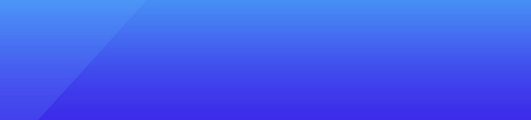
基础教程推荐
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01