boost zip_iterator and std::sort(提升 zip_iterator 和 std::sort)
问题描述
我有两个长度相同的数组 values
和 keys
.我想使用 keys
数组作为键对 values
数组进行排序.有人告诉我,boost 的 zip 迭代器是将两个数组锁定在一起并同时处理它们的正确工具.
I have two arrays values
and keys
both of the same length.
I want to sort-by-key the values
array using the keys
array as keys.
I have been told the boost's zip iterator is just the right tool for locking two arrays together and doing stuff to them at the same time.
这是我尝试使用 boost::zip_iterator 解决无法使用 gcc
编译的排序问题.有人可以帮我修复此代码吗?
Here is my attempt at using the boost::zip_iterator to solve sorting problem which fails to compile with gcc
. Can someone help me fix this code?
问题出在线路上
std::sort (boost::make_zip_iterator(keys, values), boost::make_zip_iterator(keys+N, values+N));
#include <iostream>
#include <iomanip>
#include <cstdlib>
#include <ctime>
#include <vector>
#include <algorithm>
#include <boost/iterator/zip_iterator.hpp>
#include <boost/tuple/tuple.hpp>
#include <boost/tuple/tuple_comparison.hpp>
int main(int argc, char *argv[])
{
int N=10;
int keys[N];
double values[N];
int M=100;
//Create the vectors.
for (int i = 0; i < N; ++i)
{
keys[i] = rand()%M;
values[i] = 1.0*rand()/RAND_MAX;
}
//Now we use the boost zip iterator to zip the two vectors and sort them "simulatneously"
//I want to sort-by-key the keys and values arrays
std::sort ( boost::make_zip_iterator( keys, values ),
boost::make_zip_iterator( keys+N , values+N )
);
//The values array and the corresponding keys in ascending order.
for (int i = 0; i < N; ++i)
{
std::cout << keys[i] << " " << values[i] << std::endl;
}
return 0;
}
注意:编译错误信息
g++ -g -Wall boost_test.cpp
boost_test.cpp: In function ‘int main(int, char**)’:
boost_test.cpp:37:56: error: no matching function for call to ‘make_zip_iterator(int [(((unsigned int)(((int)N) + -0x00000000000000001)) + 1)], double [(((unsigned int)(((int)N) + -0x00000000000000001)) + 1)])’
boost_test.cpp:38:64: error: no matching function for call to ‘make_zip_iterator(int*, double*)’
推荐答案
你不能对一对 zip_iterator 进行排序.
You can't sort a pair of zip_iterators.
首先,make_zip_iterator 将迭代器元组作为输入,因此您可以调用:
Firstly, make_zip_iterator takes a tuple of iterators as input, so you could call:
boost::make_zip_iterator(boost::make_tuple( ... ))
但这也不会编译,因为 keys
和 keys+N
没有相同的类型.我们需要强制 keys
成为一个指针:
but that won't compile either, because keys
and keys+N
doesn't have the same type. We need to force keys
to become a pointer:
std::sort(boost::make_zip_iterator(boost::make_tuple(+keys, +values)),
boost::make_zip_iterator(boost::make_tuple(keys+N, values+N)));
这会编译,但排序结果仍然是错误的,因为 zip_iterator 只对 可读迭代器,但 std::sort
也需要输入为 Writable 作为 此处描述,因此您无法使用 zip_iterator 进行排序.
this will compile, but the sorted result is still wrong, because a zip_iterator only models a Readable iterator, but std::sort
also needs the input to be Writable as described here, so you can't sort using zip_iterator.
这篇关于提升 zip_iterator 和 std::sort的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:提升 zip_iterator 和 std::sort
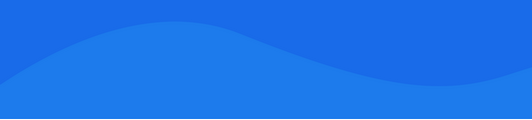
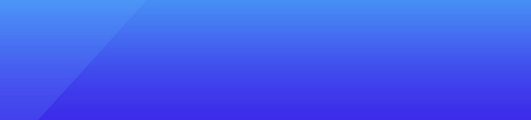
基础教程推荐
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01