C++ Converting a time string to seconds from the epoch(C++ 将时间字符串从纪元转换为秒)
问题描述
我有一个格式如下的字符串:
I have a string with the following format:
2010-11-04T23:23:01Z
2010-11-04T23:23:01Z
Z 表示时间是 UTC.
我宁愿将其存储为纪元时间,以便于比较.
The Z indicates that the time is UTC.
I would rather store this as a epoch time to make comparison easy.
推荐的方法是什么?
目前(经过快速搜索)最简单的算法是:
Currently (after a quck search) the simplist algorithm is:
1: <Convert string to struct_tm: by manually parsing string>
2: Use mktime() to convert struct_tm to epoch time.
// Problem here is that mktime uses local time not UTC time.
推荐答案
使用 C++11 功能,我们现在可以使用流来解析时间:
Using C++11 functionality we can now use streams to parse times:
iomanip std::get_time
将根据一组格式参数转换一个字符串,并将它们转换为 struct tz
对象.
The iomanip std::get_time
will convert a string based on a set of format parameters and convert them into a struct tz
object.
然后您可以使用 std::mktime()
将其转换为纪元值.
You can then use std::mktime()
to convert this into an epoch value.
#include <iostream>
#include <sstream>
#include <locale>
#include <iomanip>
int main()
{
std::tm t = {};
std::istringstream ss("2010-11-04T23:23:01Z");
if (ss >> std::get_time(&t, "%Y-%m-%dT%H:%M:%S"))
{
std::cout << std::put_time(&t, "%c") << "
"
<< std::mktime(&t) << "
";
}
else
{
std::cout << "Parse failed
";
}
return 0;
}
这篇关于C++ 将时间字符串从纪元转换为秒的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:C++ 将时间字符串从纪元转换为秒
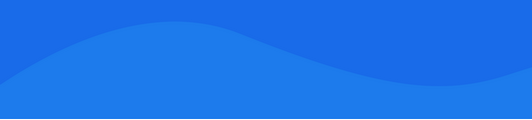
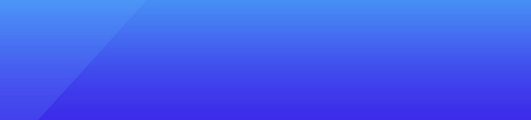
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07