Using Struct Stat()(使用 Struct Stat())
问题描述
我正在尝试弄清楚如何使用 stat() 来捕获有关文件的信息.我需要的是能够打印有关文件的多个信息字段.所以..
I'm trying to figure out how exactly to use stat() to capture information about a file. What I need is to be able to print several fields of information about a file. So..
#include <iostream>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
using namespace std;
int main() {
struct stat buf;
stat("file",&buf);
...
cout << st_dev << endl;
cout << st_ino << endl;
cout << st_mode << endl;
cout << st_nlink << endl;
cout << st_uid << endl;
cout << st_gid << endl;
cout << st_rdev << endl;
cout << st_size << endl;
cout << st_blksize << endl;
cout << st_blocks << endl;
cout << st_atime << endl;
cout << st_mtime << endl;
cout << st_ctime << endl;
...
}
我对如何做到这一点感到非常困惑.为什么 &buf 是 stat 的参数?我不在乎将这些信息存储在内存中,我只需要我的 c++ 程序中的输出字段.如何访问结构中包含的信息?buf 实际上应该包含从 stat() 返回的信息吗?
I'm thoroughly confused about how to do this. Why is &buf a parameter to stat? I don't care about storing this information in memory, I just need the outputted fields within my c++ program. How do I access the information contained in the struct? Is buf actually supposed to contain the returned information from stat()?
推荐答案
是的,buf
在这里用作输出参数.结果保存在buf
中,stat
的返回值是一个错误码,表示stat
操作是成功还是失败.
Yes, buf
is being used here as an out-parameter. The results are stored in buf
and the return value of stat
is an error code indicating if the stat
operation succeeded or failed.
这样做是因为 stat
是一个 POSIX 函数,为 C 设计,它不支持像异常这样的带外错误报告机制.如果 stat
返回 一个结构体,那么它就无法指示错误.使用这个 out-parameter 方法还允许调用者选择他们想要存储结果的位置,但这是次要功能.传递普通局部变量的地址完全没问题,就像你在这里做的一样.
It is done this way because stat
is a POSIX function, designed for C, which does not support out-of-band error reporting mechanisms like exceptions. If stat
returned a struct, then it would have no way to indicate errors. Using this out-parameter method also allows the caller to choose where they want to store the results, but that's a secondary feature. It's perfectly fine to pass the address of a normal local variable, just like you have done here.
您可以像访问任何其他对象一样访问结构体的字段.我想你至少熟悉对象符号?例如.buf.st_dev
访问名为 buf
的 stat
结构中的 st_dev
字段.所以:
You access the fields of a struct like you would any other object. I presume you are at least familar with object notation? E.g. the st_dev
field within the stat
struct called buf
is accessed by buf.st_dev
. So:
cout << buf.st_dev << endl;
等
这篇关于使用 Struct Stat()的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用 Struct Stat()
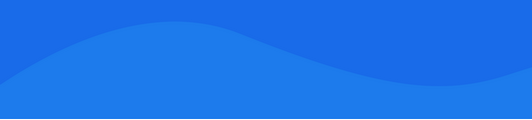
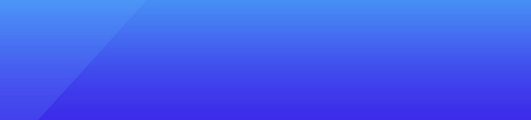
基础教程推荐
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 从 std::cin 读取密码 2021-01-01