What is the difference between static_cast and reinterpret_cast?(static_cast 和 reinterpret_cast 有什么区别?)
问题描述
可能的重复:
什么时候应该使用static_cast、dynamic_cast和reinterpret_cast?
我在 c++ 中使用 c 函数,其中在 c 中作为 void 类型参数传递的结构直接存储相同的结构类型.
I'm using c function in c++, where a structure passed as a void type argument in c is directly stored that same structure type.
例如在 C 中.
void getdata(void *data){
Testitem *ti=data;//Testitem is of struct type.
}
为了在 C++ 中做同样的事情,我使用 static_cast:
to do the same in c++ i use static_cast:
void foo::getdata(void *data){
Testitem *ti = static_cast<Testitem*>(data);
}
当我使用 reinterpret_cast
它做同样的工作,转换结构
and when i use reinterpret_cast
it does the same job, casting the struct
当我使用 Testitem *it=(Testitem *)data;
这也做同样的事情.但是使用这三个对结构有什么影响.
this does the same thing too. But how is the structure gets affected by using the three of them.
推荐答案
static_cast
是从一种类型到另一种类型的转换,它(直觉上)是在某些情况下可以成功并有意义的转换在没有危险演员的情况下.例如,您可以将 static_cast
void*
转换为 int*
,因为 void*
实际上可能指向int*
或 int
到 char
,因为这样的转换是有意义的.但是,您不能将 static_cast
int*
转换为 double*
,因为这种转换仅在 int*
以某种方式被修改为指向 double*
.
A static_cast
is a cast from one type to another that (intuitively) is a cast that could under some circumstance succeed and be meaningful in the absence of a dangerous cast. For example, you can static_cast
a void*
to an int*
, since the void*
might actually point at an int*
, or an int
to a char
, since such a conversion is meaningful. However, you cannot static_cast
an int*
to a double*
, since this conversion only makes sense if the int*
has somehow been mangled to point at a double*
.
A reinterpret_cast
是表示不安全转换的强制转换,该转换可能将一个值的位重新解释为另一个值的位.例如,将 int*
转换为 double*
对 reinterpret_cast
是合法的,尽管结果未指定.类似地,使用 reinterpret_cast
将 int
转换为 void*
是完全合法的,尽管它不安全.
A reinterpret_cast
is a cast that represents an unsafe conversion that might reinterpret the bits of one value as the bits of another value. For example, casting an int*
to a double*
is legal with a reinterpret_cast
, though the result is unspecified. Similarly, casting an int
to a void*
is perfectly legal with reinterpret_cast
, though it's unsafe.
static_cast
和 reinterpret_cast
都不能从某些东西中删除 const
.您不能使用这些转换中的任何一个将 const int*
转换为 int*
.为此,您将使用 const_cast
.
Neither static_cast
nor reinterpret_cast
can remove const
from something. You cannot cast a const int*
to an int*
using either of these casts. For this, you would use a const_cast
.
(T)
形式的 C 样式转换被定义为尝试执行 static_cast
,如果可能的话,退回到 reinterpret_cast
> 如果这不起作用.如果绝对必须,它也会应用 const_cast
.
A C-style cast of the form (T)
is defined as trying to do a static_cast
if possible, falling back on a reinterpret_cast
if that doesn't work. It also will apply a const_cast
if it absolutely must.
一般来说,你应该总是喜欢使用 static_cast
来进行安全转换.如果您不小心尝试执行未明确定义的强制转换,那么编译器将报告错误.仅当您所做的确实改变机器中某些位的解释时才使用 reinterpret_cast
,并且仅当您愿意冒险执行 reinterpret_cast<时才使用 C 风格的强制转换/代码>.在您的情况下,您应该使用
static_cast
,因为在某些情况下 void*
的向下转换是明确定义的.
In general, you should always prefer static_cast
for casting that should be safe. If you accidentally try doing a cast that isn't well-defined, then the compiler will report an error. Only use reinterpret_cast
if what you're doing really is changing the interpretation of some bits in the machine, and only use a C-style cast if you're willing to risk doing a reinterpret_cast
. In your case, you should use the static_cast
, since the downcast from the void*
is well-defined in some circumstances.
这篇关于static_cast 和 reinterpret_cast 有什么区别?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:static_cast 和 reinterpret_cast 有什么区别?
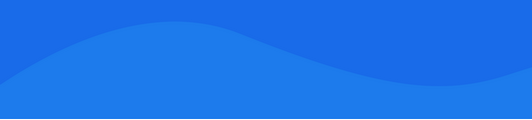
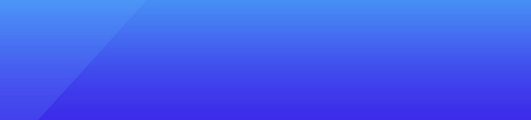
基础教程推荐
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 为什么语句不能出现在命名空间范围内? 2021-01-01