What is Proxy Class in C++(什么是 C++ 中的代理类)
问题描述
什么是 C++ 中的代理类?为什么创建它以及它的用处是什么?
What is a Proxy Class in C++? Why it is created and where it is useful?
推荐答案
代理是为另一个类提供修改后的接口的类.
A proxy is a class that provides a modified interface to another class.
这是一个例子 - 假设我们有一个数组类,我们只想包含二进制数字(1 或 0).这是第一次尝试:
Here is an example - suppose we have an array class that we only want to contain binary digits (1 or 0). Here is a first try:
struct array1 {
int mArray[10];
int & operator[](int i) {
/// what to put here
}
}; `
我们希望 operator[]
在我们说类似 a[1] = 42
时抛出,但这是不可能的,因为该操作符只能看到数组,而不是存储的值.
We want operator[]
to throw if we say something like a[1] = 42
, but that isn't possible because that operator only sees the index of the array, not the value being stored.
我们可以使用代理解决这个问题:
We can solve this using a proxy:
#include <iostream>
using namespace std;
struct aproxy {
aproxy(int& r) : mPtr(&r) {}
void operator = (int n) {
if (n > 1 || n < 0) {
throw "not binary digit";
}
*mPtr = n;
}
int * mPtr;
};
struct array {
int mArray[10];
aproxy operator[](int i) {
return aproxy(mArray[i]);
}
};
int main() {
try {
array a;
a[0] = 1; // ok
a[0] = 42; // throws exception
}
catch (const char * e) {
cout << e << endl;
}
}
代理类现在检查二进制数字,我们让数组的 operator[]
返回代理的一个实例,该实例对数组的内部具有有限的访问权限.
The proxy class now does our checking for a binary digit and we make the array's operator[]
return an instance of the proxy which has limited access to the array's internals.
这篇关于什么是 C++ 中的代理类的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:什么是 C++ 中的代理类
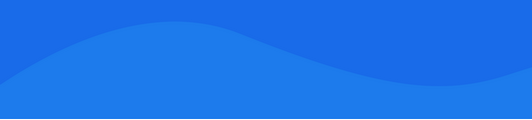
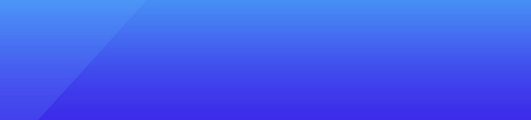
基础教程推荐
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01