The easiest way to read formatted input in C++?(在 C++ 中读取格式化输入的最简单方法?)
问题描述
有没有办法读取这样的格式化字符串,例如:48754+7812=Abcs
.
Is there any way to read a formatted string like this, for example :48754+7812=Abcs
.
假设我有三个字符串 X、Y 和 Z,我想要
Let's say I have three stringz X,Y and Z, and I want
X = 48754
Y = 7812
Z = Abcs
两个数字的大小和字符串的长度可能会有所不同,所以我不想使用 substring()
或类似的东西.
The size of the two numbers and the length of the string may vary, so I dont want to use substring()
or anything like that.
是否可以给C++这样的参数
Is it possible to give C++ a parameter like this
":#####..+####..=SSS.."
所以它直接知道发生了什么?
so it knows directly what's going on?
推荐答案
一种可能性是 boost::split()
,它允许指定多个分隔符并且不需要输入大小的先验知识:
A possibility is boost::split()
, which allows the specification of multiple delimiters and does not require prior knowledge of the size of the input:
#include <iostream>
#include <vector>
#include <string>
#include <boost/algorithm/string.hpp>
#include <boost/algorithm/string/split.hpp>
int main()
{
std::vector<std::string> tokens;
std::string s(":48754+7812=Abcs");
boost::split(tokens, s, boost::is_any_of(":+="));
// "48754" == tokens[0]
// "7812" == tokens[1]
// "Abcs" == tokens[2]
return 0;
}
或者,使用sscanf()
:
#include <iostream>
#include <cstdio>
int main()
{
const char* s = ":48754+7812=Abcs";
int X, Y;
char Z[100];
if (3 == std::sscanf(s, ":%d+%d=%99s", &X, &Y, Z))
{
std::cout << "X=" << X << "
";
std::cout << "Y=" << Y << "
";
std::cout << "Z=" << Z << "
";
}
return 0;
}
然而,这里的限制是字符串的最大长度 (Z
) 必须在解析输入之前确定.
However, the limitiation here is that the maximum length of the string (Z
) must be decided before parsing the input.
这篇关于在 C++ 中读取格式化输入的最简单方法?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 C++ 中读取格式化输入的最简单方法?
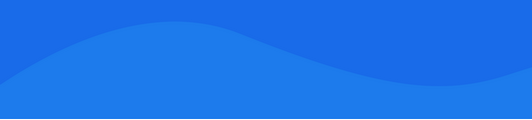
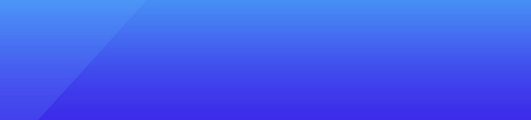
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 从 std::cin 读取密码 2021-01-01