Serializing a class which contains a std::string(序列化包含 std::string 的类)
问题描述
我不是 C++ 专家,但过去我已经将事情序列化了几次.不幸的是,这次我试图序列化一个包含 std::string 的类,我理解这很像序列化一个指针.
I'm not a c++ expert but I've serialized things a couple of times in the past. Unfortunately this time I'm trying to serialize a class which contains a std::string, which I understand is pretty much like serializing a pointer.
我可以将类写出到文件中,然后再读回.所有 int 字段都很好,但 std::string 字段给出了地址越界"错误,大概是因为它指向不再存在的数据.
I can write out the class to a file and read it back in again. All int fields are fine but the std::string field gives an "address out of bounds" error, presumably because it points to data which is no longer there.
对此有标准的解决方法吗?我不想回到字符数组,但至少我知道它们在这种情况下工作.如有必要,我可以提供代码,但我希望我已经很好地解释了我的问题.
Is there a standard workaround for this? I don't want to go back to char arrays but at least I know they work in this situation. I can provide code if necessary but I'm hoping I've explained my problem well.
我通过将类转换为 char* 并使用 fstream 将其写入文件来进行序列化.阅读当然正好相反.
I'm serializing by casting the class to a char* and writing it to a file with fstream. Reading of course is just the reverse.
推荐答案
我通过将类转换为 char* 并将其写入文件与 fstream.阅读当然正好相反.
I'm serializing by casting the class to a char* and writing it to a file with fstream. Reading of course is just the reverse.
不幸的是,这仅在不涉及指针时才有效.你可能想给你的类 void MyClass::serialize(std::ostream)
和 void MyClass::deserialize(std::ifstream)
,然后调用它们.对于这种情况,您需要
Unfortunately, this only works as long as there are no pointers involved. You might want to give your classes void MyClass::serialize(std::ostream)
and void MyClass::deserialize(std::ifstream)
, and call those. For this case, you'd want
std::ostream& MyClass::serialize(std::ostream &out) const {
out << height;
out << ',' //number seperator
out << width;
out << ',' //number seperator
out << name.size(); //serialize size of string
out << ',' //number seperator
out << name; //serialize characters of string
return out;
}
std::istream& MyClass::deserialize(std::istream &in) {
if (in) {
int len=0;
char comma;
in >> height;
in >> comma; //read in the seperator
in >> width;
in >> comma; //read in the seperator
in >> len; //deserialize size of string
in >> comma; //read in the seperator
if (in && len) {
std::vector<char> tmp(len);
in.read(tmp.data() , len); //deserialize characters of string
name.assign(tmp.data(), len);
}
}
return in;
}
您可能还想重载流运算符以便于使用.
You may also want to overload the stream operators for easier use.
std::ostream &operator<<(std::ostream& out, const MyClass &obj)
{obj.serialize(out); return out;}
std::istream &operator>>(std::istream& in, MyClass &obj)
{obj.deserialize(in); return in;}
这篇关于序列化包含 std::string 的类的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:序列化包含 std::string 的类
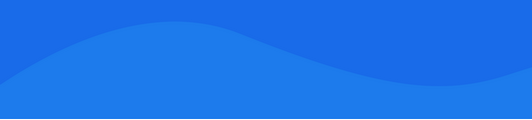
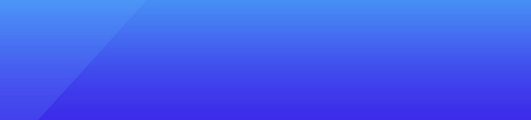
基础教程推荐
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01