Sorting vector of pointers(指针的排序向量)
问题描述
我在尝试对指针向量进行排序时遇到了一些麻烦.
I'm having a little trouble trying to sort a vector of pointers.
这是我目前所做的:
class Node
{
private:
vector <Node*> _children;
string _data;
...
public:
void Node::add_child(Node* child)
{
...
sort(_children.begin(), _children.end());
}
bool Node::operator<(const Node& node)
{
return (this->_data.compare(node._data) == -1);
}
};
我的小于运算符有效,如果我这样写:
My less-than operator works, if I write like this:
Node* root = new Node("abc");
Node* n = new Node("def");
cout << (*root<*n) << endl;
为什么 sort 从不调用操作符??任何帮助,将不胜感激!谢谢.
Why does sort never call the operator?? Any help would be appreciated! Thanks.
马绍夫
推荐答案
因为您对指针值进行排序,而不是对它们指向的 Node
进行排序.
Because you sort the pointer values, not the Node
s they point to.
您可以使用 std::sort
的第三个参数 指定自定义比较器的算法.
You can use the third argument of the std::sort
algorithm to specify a custom comparator.
例如:
bool comparePtrToNode(Node* a, Node* b) { return (*a < *b); }
std::sort(_children.begin(), _children.end(), comparePtrToNode);
(请注意,此代码只是一个指示 - 您必须在需要的地方添加额外的安全检查)
(note that this code is just an indication - you'll have to add extra safety checks where needed)
这篇关于指针的排序向量的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:指针的排序向量
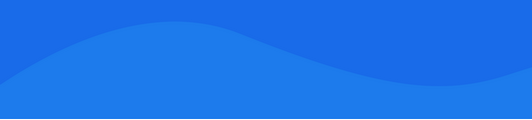
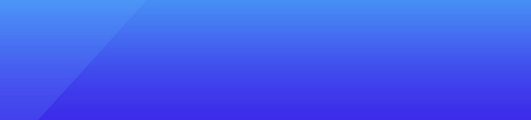
基础教程推荐
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 使用从字符串中提取的参数调用函数 2022-01-01