The correct way to initialize a dynamic pointer to a multidimensional array?(初始化指向多维数组的动态指针的正确方法?)
问题描述
当我将动态指针范围扩展到 2 维和更高维度时,我对动态指针的处理一直很糟糕.例如,我想要一个指向二维数组的指针.我知道:
I've been having bad luck with with dynamic pointers when I range them to 2 dimensions and higher. For example I want a pointer to a 2D array. I know that:
int A[3][4];
int (*P)[4] = A;
完全合法(即使我不完全理解为什么).考虑到:
Is completely legit (even if I don't completely understand why). Taking into consideration that:
int *P = new int[4];
作品,我想象的:
int **P = new int[5][7];
也可以,但不行.此代码说明错误:
Would also work, but it's not. This code states the error:
Error: A value of type "(*)[7]" cannot be used to initialize an entity of
type "int **"
通过看到这一点,新部分变成了一个指向我创建的 7 个整数数组的指针:
By seeing this the new part becomes a pointer to an array of 7 integers I made:
int (*P)[4] = new int[7][4];
这确实有效,但这不是我想要完成的.通过这样做,我被限制为至少对任何后续维度使用一个常量值,但我希望它在运行时完全定义,因此是动态的".
And this does work but it's not what I want to accomplish. By doing it like that I'm limited to at least using a constant value for any subsequent dimension, but I want it to be fully defined at run time and therefore "dynamic".
我怎样才能让这个多维指针工作??
How could I go and make this multidimensional pointer work??
推荐答案
让我们从一些基本的例子开始.
Let's start with some basic examples.
当你说int *P = new int[4];
new int[4];
调用 operator new function()- 为 4 个整数分配内存.
- 返回对此内存的引用.
要绑定这个引用,你需要有与返回引用相同类型的指针,所以你这样做
new int[4];
calls operator new function()- allocates a memory for 4 integers.
- returns a reference to this memory.
to bind this reference, you need to have same type of pointer as that of return reference so you do
int *P = new int[4]; // As you created an array of integer
// you should assign it to a pointer-to-integer
对于多维数组,您需要分配一个指针数组,然后用指向数组的指针填充该数组,如下所示:
For a multi-idimensional array, you need to allocate an array of pointers, then fill that array with pointers to arrays, like this:
int **p;
p = new int*[5]; // dynamic `array (size 5) of pointers to int`
for (int i = 0; i < 5; ++i) {
p[i] = new int[10];
// each i-th pointer is now pointing to dynamic array (size 10)
// of actual int values
}
这是它的样子:
对于一维数组,
For one dimensional array,
// need to use the delete[] operator because we used the new[] operator
delete[] p; //free memory pointed by p;`
对于二维数组,
For 2d Array,
// need to use the delete[] operator because we used the new[] operator
for(int i = 0; i < 5; ++i){
delete[] p[i];//deletes an inner array of integer;
}
delete[] p; //delete pointer holding array of pointers;
避免内存泄漏和悬空指针!
这篇关于初始化指向多维数组的动态指针的正确方法?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:初始化指向多维数组的动态指针的正确方法?
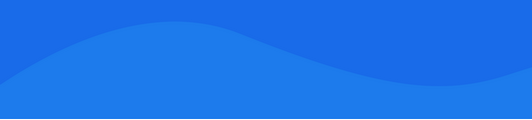
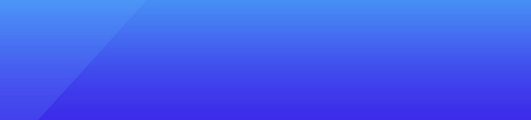
基础教程推荐
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01