Multiplication of each matrix column by each vector element using Eigen C++ Library(使用 Eigen C++ 库将每个矩阵列与每个向量元素相乘)
问题描述
我需要使用 Eigen C++ 库 将每个矩阵列乘以每个向量元素.我试过 colwise 没有成功.
I need to multiply each matrix column by each vector element using Eigen C++ library. I tried colwise without success.
示例数据:
Eigen::Matrix3Xf A(3,2); //3x2
A << 1 2,
2 2,
3 5;
Eigen::Vector3f V = Eigen::Vector3f(2, 3);
//Expected result
C = A.colwise()*V;
//C
//2 6,
//4 6,
//6 15
//this means C 1st col by V first element and C 2nd col by V 2nd element.
矩阵 A 可以有 3xN 和 V Nx1.含义 (cols x rowls).
Matrix A can have 3xN and V Nx1. Meaning (cols x rowls).
推荐答案
我会这样做:
Eigen::Matrix3Xf A(3, 2); // 3x2
A << 1, 2, 2, 2, 3, 5;
Eigen::Vector3f V = Eigen::Vector3f(1, 2, 3);
const Eigen::Matrix3Xf C = A.array().colwise() * V.array();
std::cout << C << std::endl;
示例输出:
1 2
4 4
9 15
说明
你很接近,诀窍是使用 .array()
来做广播乘法.
colwiseReturnType
没有 .array()
方法,所以我们必须在 A 的数组视图上做我们的 colwise 恶作剧.
colwiseReturnType
doesn't have a .array()
method, so we have to do our colwise shenanigans on the array view of A.
如果你想计算两个向量的元素乘积(最酷的酷猫称之为 Hadamard 产品),你可以做
If you want to compute the element-wise product of two vectors (The coolest of cool cats call this the Hadamard Product), you can do
Eigen::Vector3f a = ...;
Eigen::Vector3f b = ...;
Eigen::Vector3f elementwise_product = a.array() * b.array();
以上代码以列方式执行的操作.
Which is what the above code is doing, in a columnwise fashion.
要解决行情况,您可以使用 .rowwise()
,并且您需要一个额外的 transpose()
以使事情适合
To address the row case, you can use .rowwise()
, and you'll need an extra transpose()
to make things fit
Eigen::Matrix<float, 3, 2> A; // 3x2
A << 1, 2, 2, 2, 3, 5;
Eigen::Vector2f V = Eigen::Vector2f(2, 3);
// Expected result
Eigen::Matrix<float, 3, 2> C = A.array().rowwise() * V.transpose().array();
std::cout << C << std::endl;
示例输出:
2 6
4 6
6 15
这篇关于使用 Eigen C++ 库将每个矩阵列与每个向量元素相乘的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用 Eigen C++ 库将每个矩阵列与每个向量元素相乘
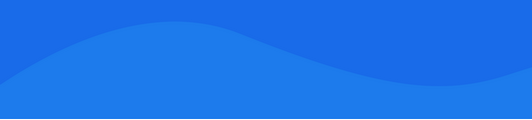
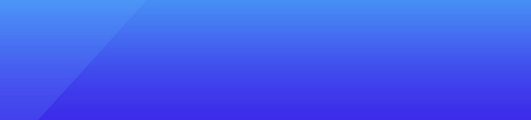
基础教程推荐
- Windows Media Foundation 录制音频 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 使用从字符串中提取的参数调用函数 2022-01-01