Range based for-loop on array passed to non-main function(传递给非主函数的数组上基于范围的 for 循环)
问题描述
当我尝试在 gcc 4.8.2 中编译以下代码时,出现以下错误:
<块引用>test.cc: 在函数‘void foo(int*)’中:test.cc:15:16: 错误:没有匹配的函数调用‘begin(int*&)’for (int i : bar) {^
以及来自模板库深处的其他一些人.
#include 使用命名空间标准;void foo(int*);int main() {int bar[3] = {1,2,3};for (int i : bar) {cout<<我<<结束;}富(酒吧);}void foo(int* bar) {for (int i : bar) {cout<<我<<结束;}}
如果我重新定义 foo
以使用索引的 for 循环,那么代码会编译并按预期运行.此外,如果我将基于范围的输出循环移动到 main
中,我也会得到预期的行为.
如何将数组 bar
传递给 foo
使其能够在其上执行基于范围的 for 循环?
随着数组衰减变成一个指针,你'丢失了一条重要信息:它的大小.
使用数组引用,您的基于范围的循环可以工作:
void foo(int (&bar)[3]);int main() {int bar[3] = {1,2,3};for (int i : bar) {cout<<我<<结束;}富(酒吧);}void foo(int (&bar)[3]) {for (int i : bar) {cout<<我<<结束;}}
或者,以通用方式(即在函数签名中不指定数组大小),
模板void foo(int (&bar)[array_size]) {for (int i : bar) {cout<<我<<结束;}}
试试
When I try to compile the following code in gcc 4.8.2, I get the following error:
test.cc: In function ‘void foo(int*)’: test.cc:15:16: error: no matching function for call to ‘begin(int*&)’ for (int i : bar) { ^
Along with a bunch of others from deeper in the template library.
#include <iostream>
using namespace std;
void foo(int*);
int main() {
int bar[3] = {1,2,3};
for (int i : bar) {
cout << i << endl;
}
foo(bar);
}
void foo(int* bar) {
for (int i : bar) {
cout << i << endl;
}
}
If I redefine foo
to use an indexed for loop, then the code compiles and behaves as expected. Also, if I move the range-based output loop into main
, I get the expected behaviour as well.
How do I pass the array bar
to foo
in such a way that it is capable of executing a range-based for-loop on it?
With the array decaying into a pointer you're losing one important piece of information: its size.
With an array reference your range based loop works:
void foo(int (&bar)[3]);
int main() {
int bar[3] = {1,2,3};
for (int i : bar) {
cout << i << endl;
}
foo(bar);
}
void foo(int (&bar)[3]) {
for (int i : bar) {
cout << i << endl;
}
}
or, in a generic fashion (i.e. without specifying the array size in the function signature),
template <std::size_t array_size>
void foo(int (&bar)[array_size]) {
for (int i : bar) {
cout << i << endl;
}
}
Try it out
这篇关于传递给非主函数的数组上基于范围的 for 循环的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:传递给非主函数的数组上基于范围的 for 循环
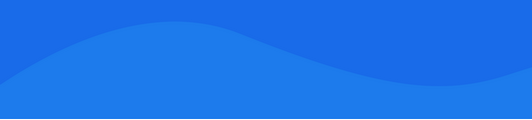
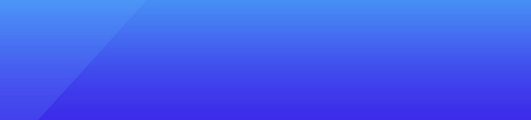
基础教程推荐
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- C++,'if' 表达式中的变量声明 2021-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01