How to use std::sort with a vector of structures and compare function?(如何将 std::sort 与结构向量和比较函数一起使用?)
问题描述
感谢C 中的解决方案,现在我想在 C++ 中使用 std::sort 和 vector 来实现这一点:
Thanks for a solution in C, now I would like to achieve this in C++ using std::sort and vector:
typedef struct
{
double x;
double y;
double alfa;
} pkt;
向量<包>wektor;
使用 push_back() 填充;比较函数:
vector< pkt > wektor;
filled up using push_back(); compare function:
int porownaj(const void *p_a, const void *p_b)
{
pkt *pkt_a = (pkt *) p_a;
pkt *pkt_b = (pkt *) p_b;
if (pkt_a->alfa > pkt_b->alfa) return 1;
if (pkt_a->alfa < pkt_b->alfa) return -1;
if (pkt_a->x > pkt_b->x) return 1;
if (pkt_a->x < pkt_b->x) return -1;
return 0;
}
sort(wektor.begin(), wektor.end(), porownaj); // this makes loads of errors on compile time
要纠正什么?在这种情况下如何正确使用 std::sort?
What is to correct? How to use properly std::sort in that case?
推荐答案
std::sort
采用与 qsort
中使用的比较函数不同的比较函数.该函数不返回 –1、0 或 1,而是返回一个 bool
值,指示第一个元素是否小于第二个元素.
std::sort
takes a different compare function from that used in qsort
. Instead of returning –1, 0 or 1, this function is expected to return a bool
value indicating whether the first element is less than the second.
您有两种可能性:为您的对象实现 operator <
;在这种情况下,没有第三个参数的默认 sort
调用将起作用;或者你可以重写上面的函数来完成同样的事情.
You have two possibilites: implement operator <
for your objects; in that case, the default sort
invocation without a third argument will work; or you can rewrite your above function to accomplish the same thing.
请注意,您必须在参数中使用强类型.
Notice that you have to use strong typing in the arguments.
另外,这里根本不使用函数也不错.相反,使用函数对象.这些受益于内联.
Additionally, it's good not to use a function here at all. Instead, use a function object. These benefit from inlining.
struct pkt_less {
bool operator ()(pkt const& a, pkt const& b) const {
if (a.alfa < b.alfa) return true;
if (a.alfa > b.alfa) return false;
if (a.x < b.x) return true;
if (a.x > b.x) return false;
return false;
}
};
// Usage:
sort(wektor.begin(), wektor.end(), pkt_less());
这篇关于如何将 std::sort 与结构向量和比较函数一起使用?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何将 std::sort 与结构向量和比较函数一起使用
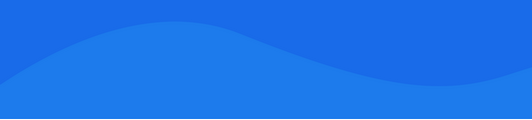
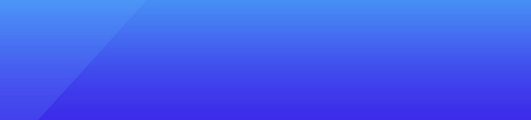
基础教程推荐
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17