Sorting std::map using value(使用值对 std::map 进行排序)
问题描述
我需要按值而不是键对 std::map
进行排序.有什么简单的方法吗?
I need to sort an std::map
by value rather than by key. Is there an easy way to do it?
我从以下线程中得到了一个解决方案:
std::map 按数据排序?
有更好的解决方案吗?
I got one solution from the follwing thread:
std::map sort by data?
Is there a better solution?
map<long, double> testMap;
// some code to generate the values in the map.
sort(testMap.begin(), testMap.end()); // is there any function like this to sort the map?
推荐答案
即使正确答案已经发布,我还是想添加一个演示,说明如何干净利落地做到这一点:
Even though correct answers have already been posted, I thought I'd add a demo of how you can do this cleanly:
template<typename A, typename B>
std::pair<B,A> flip_pair(const std::pair<A,B> &p)
{
return std::pair<B,A>(p.second, p.first);
}
template<typename A, typename B>
std::multimap<B,A> flip_map(const std::map<A,B> &src)
{
std::multimap<B,A> dst;
std::transform(src.begin(), src.end(), std::inserter(dst, dst.begin()),
flip_pair<A,B>);
return dst;
}
int main(void)
{
std::map<int, double> src;
...
std::multimap<double, int> dst = flip_map(src);
// dst is now sorted by what used to be the value in src!
}
<小时>
通用关联源(需要 C++11)
如果您为源关联容器使用 std::map
的替代品(例如 std::unordered_map
),您可以编写一个单独的重载,但最终动作还是一样的,所以使用可变参数模板的广义关联容器可以用于任一映射构造:
If you're using an alternate to std::map
for the source associative container (such as std::unordered_map
), you could code a separate overload, but in the end the action is still the same, so a generalized associative container using variadic templates can be used for either mapping construct:
// flips an associative container of A,B pairs to B,A pairs
template<typename A, typename B, template<class,class,class...> class M, class... Args>
std::multimap<B,A> flip_map(const M<A,B,Args...> &src)
{
std::multimap<B,A> dst;
std::transform(src.begin(), src.end(),
std::inserter(dst, dst.begin()),
flip_pair<A,B>);
return dst;
}
这对于作为翻转源的 std::map
和 std::unordered_map
都有效.
This will work for both std::map
and std::unordered_map
as the source of the flip.
这篇关于使用值对 std::map 进行排序的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用值对 std::map 进行排序
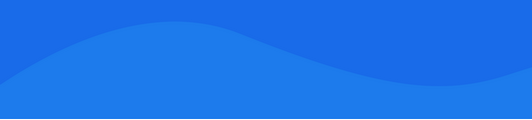
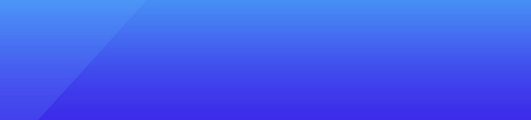
基础教程推荐
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04