Convert RGB to Black amp; White in OpenCV(将 RGB 转换为黑色 amp;OpenCV 中的白色)
问题描述
我想知道如何将 RGB 图像转换为黑色 &白色(二进制)图像.
I would like to know how to convert an RGB image into a black & white (binary) image.
转换后,如何将修改后的图像保存到磁盘?
After conversion, how can I save the modified image to disk?
推荐答案
AFAIK,你必须把它转换成灰度,然后阈值转换成二进制.
AFAIK, you have to convert it to grayscale and then threshold it to binary.
1.将图像读取为灰度图像如果您是从磁盘读取 RGB 图像,那么您可以直接将其作为灰度图像读取,如下所示:
1. Read the image as a grayscale image If you're reading the RGB image from disk, then you can directly read it as a grayscale image, like this:
// C
IplImage* im_gray = cvLoadImage("image.jpg",CV_LOAD_IMAGE_GRAYSCALE);
// C++ (OpenCV 2.0)
Mat im_gray = imread("image.jpg",CV_LOAD_IMAGE_GRAYSCALE);
2.将RGB图像im_rgb
转换为灰度图像:否则,您必须将之前获得的RGB图像转换为灰度图像
2. Convert an RGB image im_rgb
into a grayscale image: Otherwise, you'll have to convert the previously obtained RGB image into a grayscale image
// C
IplImage *im_rgb = cvLoadImage("image.jpg");
IplImage *im_gray = cvCreateImage(cvGetSize(im_rgb),IPL_DEPTH_8U,1);
cvCvtColor(im_rgb,im_gray,CV_RGB2GRAY);
// C++
Mat im_rgb = imread("image.jpg");
Mat im_gray;
cvtColor(im_rgb,im_gray,CV_RGB2GRAY);
3.转换为二进制您可以使用自适应阈值或固定级别阈值 将您的灰度图像转换为二值图像.
3. Convert to binary You can use adaptive thresholding or fixed-level thresholding to convert your grayscale image to a binary image.
例如在 C 中,您可以执行以下操作(您也可以在 C++ 中使用 Mat 和相应的函数执行相同的操作):
E.g. in C you can do the following (you can also do the same in C++ with Mat and the corresponding functions):
// C
IplImage* im_bw = cvCreateImage(cvGetSize(im_gray),IPL_DEPTH_8U,1);
cvThreshold(im_gray, im_bw, 128, 255, CV_THRESH_BINARY | CV_THRESH_OTSU);
// C++
Mat img_bw = im_gray > 128;
在上面的例子中,128 是阈值.
In the above example, 128 is the threshold.
4.保存到磁盘
// C
cvSaveImage("image_bw.jpg",img_bw);
// C++
imwrite("image_bw.jpg", img_bw);
这篇关于将 RGB 转换为黑色 &OpenCV 中的白色的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:将 RGB 转换为黑色 &OpenCV 中的白色
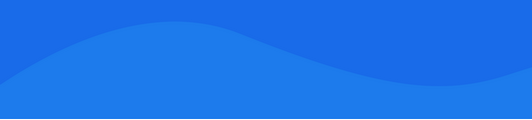
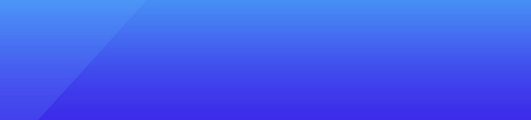
基础教程推荐
- 设计字符串本地化的最佳方法 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31