Thread safe implementation of circular buffer(循环缓冲区的线程安全实现)
问题描述
来自 boost 库的 Circular_buffer 不是线程安全的.所以我将 boost::circular_buffer 对象包装在一个类中,如下所示.线程之间的互斥是通过使用条件变量、互斥锁和锁获取/释放来实现的(我认为).这个实现线程安全吗?
Circular_buffer from boost library is not thread-safe. So I wrapped boost::circular_buffer object in a class as shown below. Mutual exclusion between the threads is achieved (I think) by using conditional variables, a mutex and a lock acquisition/release. Is this implementation thread safe?
#include <boost/thread/condition.hpp>
#include <boost/thread/mutex.hpp>
#include <boost/thread/thread.hpp>
#include <boost/circular_buffer.hpp>
// Thread safe circular buffer
template <typename T>
class circ_buffer : private boost::noncopyable
{
public:
typedef boost::mutex::scoped_lock lock;
circ_buffer() {}
circ_buffer(int n) {cb.set_capacity(n);}
void send (T imdata) {
lock lk(monitor);
cb.push_back(imdata);
buffer_not_empty.notify_one();
}
T receive() {
lock lk(monitor);
while (cb.empty())
buffer_not_empty.wait(lk);
T imdata = cb.front();
cb.pop_front();
return imdata;
}
void clear() {
lock lk(monitor);
cb.clear();
}
int size() {
lock lk(monitor);
return cb.size();
}
void set_capacity(int capacity) {
lock lk(monitor);
cb.set_capacity(capacity);
}
private:
boost::condition buffer_not_empty;
boost::mutex monitor;
boost::circular_buffer<T> cb;
};
Edit 这现在是一个模板类,它接受任何类型的对象(不仅仅是 cv::Mat
对象).
Edit This is now a template class, which accepts an object of any type (not just cv::Mat
object).
推荐答案
是.
如果您使用相同的锁锁定所有公共方法,它将是线程安全的.
Yes.
If you lock all the public methods with the same lock it will be threadsafe.
您可以考虑使用读写锁,如果您使用它可能会有更好的性能有很多并发读者.
You could consider using read-write locks, which may have better performance if you have a lot of concurrent readers.
如果你没有很多读者,它只会增加开销,但可能值得检查选项和测试.
If you don't have a lot of readers, it will just add overhead, but may be worth checking the option and testing.
这篇关于循环缓冲区的线程安全实现的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:循环缓冲区的线程安全实现
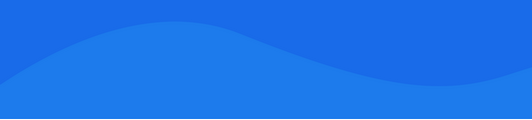
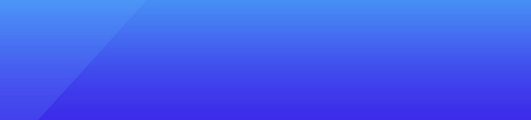
基础教程推荐
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 运算符重载的基本规则和习语是什么? 2022-10-31