problem sorting using member function as comparator(使用成员函数作为比较器进行排序的问题)
问题描述
尝试编译以下代码时出现此编译错误,我该怎么办?
trying to compile the following code I get this compile error, what can I do?
ISO C++ 禁止取地址不合格的或括号内的非静态成员函数形成一个指向成员函数的指针.
ISO C++ forbids taking the address of an unqualified or parenthesized non-static member function to form a pointer to member function.
class MyClass {
int * arr;
// other member variables
MyClass() { arr = new int[someSize]; }
doCompare( const int & i1, const int & i2 ) { // use some member variables }
doSort() { std::sort(arr,arr+someSize, &doCompare); }
};
推荐答案
doCompare
必须是 static
.如果 doCompare
需要来自 MyClass
的数据,您可以通过更改将 MyClass
变成一个比较函子:
doCompare
must be static
. If doCompare
needs data from MyClass
you could turn MyClass
into a comparison functor by changing:
doCompare( const int & i1, const int & i2 ) { // use some member variables }
进入
bool operator () ( const int & i1, const int & i2 ) { // use some member variables }
并调用:
doSort() { std::sort(arr, arr+someSize, *this); }
另外,doSort
是不是缺少返回值?
Also, isn't doSort
missing a return value?
我认为应该可以使用 std::mem_fun
和某种绑定将成员函数转换为自由函数,但目前我无法理解确切的语法.
I think it should be possible to use std::mem_fun
and some sort of binding to turn the member function into a free function, but the exact syntax evades me at the moment.
Doh,std::sort
按值获取函数,这可能是一个问题.为了解决这个问题,将函数包装在类中:
Doh, std::sort
takes the function by value which may be a problem. To get around this wrap the function inside the class:
class MyClass {
struct Less {
Less(const MyClass& c) : myClass(c) {}
bool operator () ( const int & i1, const int & i2 ) {// use 'myClass'}
MyClass& myClass;
};
doSort() { std::sort(arr, arr+someSize, Less(*this)); }
}
这篇关于使用成员函数作为比较器进行排序的问题的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用成员函数作为比较器进行排序的问题
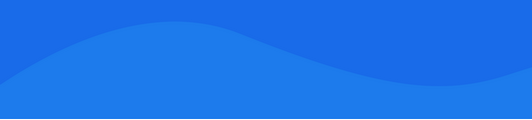
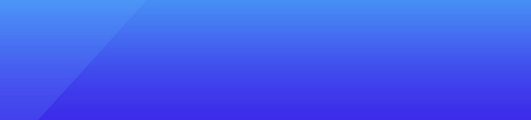
基础教程推荐
- 设计字符串本地化的最佳方法 2022-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01