Read from QTcpSocket using QDataStream(使用 QDataStream 从 QTcpSocket 读取)
问题描述
我需要通过 QTcpSocket
发送二进制数据.我正在考虑使用 QDataStream
,但我遇到了一个问题 - 如果在我尝试读取时没有数据到达,它会默默地失败.
I need to send binary data through a QTcpSocket
. I was thinking about using QDataStream
, but I've encountered a problem - it silently fails if no data has arrived at the time I try to read.
例如,如果我有这个代码:
For example if I have this code:
QString str;
stream >> str;
如果套接字中当前没有数据,它将静默失败.有没有办法让它改为阻止?
It will fail silently if no data is currently there in the socket. Is there a way to tell it to block instead?
推荐答案
我根据@Marek 的想法重新编写了代码并创建了 2 个类 - BlockReader 和 BlockWriter:
I reworked the code from @Marek's idea and created 2 classes - BlockReader and BlockWriter:
// Write block to the socket.
BlockWriter(socket).stream() << QDir("C:/Windows").entryList() << QString("Hello World!");
....
// Now read the block from the socket.
QStringList infoList;
QString s;
BlockReader(socket).stream() >> infoList >> s;
qDebug() << infoList << s;
BlockReader:
class BlockReader
{
public:
BlockReader(QIODevice *io)
{
buffer.open(QIODevice::ReadWrite);
_stream.setVersion(QDataStream::Qt_4_8);
_stream.setDevice(&buffer);
quint64 blockSize;
// Read the size.
readMax(io, sizeof(blockSize));
buffer.seek(0);
_stream >> blockSize;
// Read the rest of the data.
readMax(io, blockSize);
buffer.seek(sizeof(blockSize));
}
QDataStream& stream()
{
return _stream;
}
private:
// Blocking reads data from socket until buffer size becomes exactly n. No
// additional data is read from the socket.
void readMax(QIODevice *io, int n)
{
while (buffer.size() < n) {
if (!io->bytesAvailable()) {
io->waitForReadyRead(30000);
}
buffer.write(io->read(n - buffer.size()));
}
}
QBuffer buffer;
QDataStream _stream;
};
块写入器:
class BlockWriter
{
public:
BlockWriter(QIODevice *io)
{
buffer.open(QIODevice::WriteOnly);
this->io = io;
_stream.setVersion(QDataStream::Qt_4_8);
_stream.setDevice(&buffer);
// Placeholder for the size. We will get the value
// at the end.
_stream << quint64(0);
}
~BlockWriter()
{
// Write the real size.
_stream.device()->seek(0);
_stream << (quint64) buffer.size();
// Flush to the device.
io->write(buffer.buffer());
}
QDataStream &stream()
{
return _stream;
}
private:
QBuffer buffer;
QDataStream _stream;
QIODevice *io;
};
这篇关于使用 QDataStream 从 QTcpSocket 读取的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用 QDataStream 从 QTcpSocket 读取
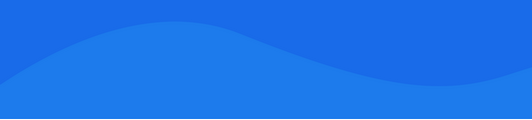
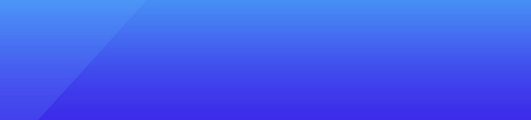
基础教程推荐
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17