Function pointer vs Function reference(函数指针与函数引用)
问题描述
在下面的代码中,函数指针和我认为的函数引用"似乎具有相同的语义:
In the code below, function-pointer and what i considered as "function-reference" seems to have identical semantics:
#include <iostream>
using std::cout;
void func(int a) {
cout << "Hello" << a << '
';
}
void func2(int a) {
cout << "Hi" << a << '
';
}
int main() {
void (& f_ref)(int) = func;
void (* f_ptr)(int) = func;
// what i expected to be, and is, correct:
f_ref(1);
(*f_ptr)(2);
// what i expected to be, and is not, wrong:
(*f_ref)(4); // i even added more stars here like (****f_ref)(4)
f_ptr(3); // everything just works!
// all 4 statements above works just fine
// the only difference i found, as one would expect:
// f_ref = func2; // ERROR: read-only reference
f_ptr = func2; // works fine!
f_ptr(5);
return 0;
}
我在 Fedora/Linux 中使用了 gcc 4.7.2 版
I used gcc version 4.7.2 in Fedora/Linux
更新
我的问题是:
为什么函数指针不需要解引用?为什么取消引用函数引用不会导致错误?- 是否在某些情况下我必须使用一种而不是另一种?
- 为什么
f_ptr = &func;
有效?既然 func 应该衰减成一个指针?
虽然f_ptr = &&func;
不起作用(从void *
隐式转换)
Why function pointer does not require dereferencing?Why dereferencing a function reference doesn't result in an error?- Is(Are) there any situation(s) where I must use one over the other?
- Why
f_ptr = &func;
works? Since func should be decayed into a pointer?
Whilef_ptr = &&func;
doesn't work (implicit conversion fromvoid *
)
推荐答案
函数和函数引用(即那些类型的 id-expressions)几乎立即衰减为函数指针,因此表达式 func
和 f_ref
在您的情况下实际上成为函数指针.如果你愿意,你也可以调用 (***func)(5)
和 (******f_ref)(6)
.
Functions and function references (i.e. id-expressions of those types) decay into function pointers almost immediately, so the expressions func
and f_ref
actually become function pointers in your case. You can also call (***func)(5)
and (******f_ref)(6)
if you like.
在您希望 &
-operator 像已应用于函数本身一样工作的情况下,最好使用函数引用,例如&func
与 &f_ref
相同,但 &f_ptr
是另一回事.
It may be preferable to use function references in cases where you want the &
-operator to work as though it had been applied to the function itself, e.g. &func
is the same as &f_ref
, but &f_ptr
is something else.
这篇关于函数指针与函数引用的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:函数指针与函数引用
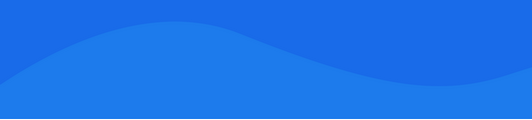
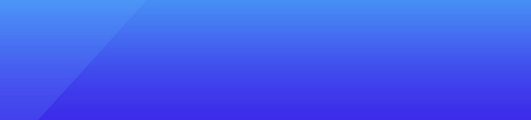
基础教程推荐
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++,'if' 表达式中的变量声明 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01