How to make thread sleep less than a millisecond on Windows(如何在 Windows 上使线程休眠少于一毫秒)
问题描述
在 Windows 上,我遇到了在 Unix 上从未遇到过的问题.这就是让线程休眠少于一毫秒的方法.在 Unix 上,您通常有多种选择(sleep、usleep 和 nanosleep)来满足您的需求.然而,在 Windows 上,只有 Sleep 以毫秒为粒度.
On Windows I have a problem I never encountered on Unix. That is how to get a thread to sleep for less than one millisecond. On Unix you typically have a number of choices (sleep, usleep and nanosleep) to fit your needs. On Windows, however, there is only Sleep with millisecond granularity.
在 Unix 上,我可以使用 select
系统调用来创建一个非常简单的微秒睡眠:
On Unix, I can use the use the select
system call to create a microsecond sleep which is pretty straightforward:
int usleep(long usec)
{
struct timeval tv;
tv.tv_sec = usec/1000000L;
tv.tv_usec = usec%1000000L;
return select(0, 0, 0, 0, &tv);
}
如何在 Windows 上实现相同的效果?
How can I achieve the same on Windows?
推荐答案
在 Windows 上使用 select
强制您包含 Winsock 库,它必须在您的应用程序中像这样初始化:
On Windows the use of select
forces you to include the Winsock library which has to be initialized like this in your application:
WORD wVersionRequested = MAKEWORD(1,0);
WSADATA wsaData;
WSAStartup(wVersionRequested, &wsaData);
然后 select 将不允许在没有任何套接字的情况下调用您,因此您必须做更多的事情来创建 microsleep 方法:
And then the select won't allow you to be called without any socket so you have to do a little more to create a microsleep method:
int usleep(long usec)
{
struct timeval tv;
fd_set dummy;
SOCKET s = socket(PF_INET, SOCK_STREAM, IPPROTO_TCP);
FD_ZERO(&dummy);
FD_SET(s, &dummy);
tv.tv_sec = usec/1000000L;
tv.tv_usec = usec%1000000L;
return select(0, 0, 0, &dummy, &tv);
}
所有这些创建的 usleep 方法在成功时返回零,错误时返回非零.
All these created usleep methods return zero when successful and non-zero for errors.
这篇关于如何在 Windows 上使线程休眠少于一毫秒的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 Windows 上使线程休眠少于一毫秒
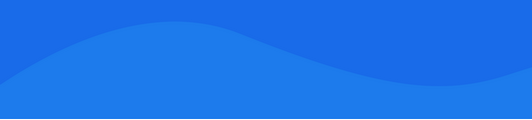
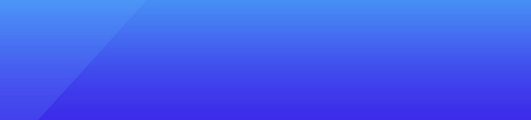
基础教程推荐
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++,'if' 表达式中的变量声明 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 设计字符串本地化的最佳方法 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01