Passing object by reference to std::thread in C++11(在 C++11 中通过引用 std::thread 传递对象)
问题描述
为什么在创建 std::thread
时不能通过引用传递对象?
Why can't you pass an object by reference when creating a std::thread
?
例如下面的代码段给出了一个编译错误:
For example the following snippit gives a compile error:
#include <iostream>
#include <thread>
using namespace std;
static void SimpleThread(int& a) // compile error
//static void SimpleThread(int a) // OK
{
cout << __PRETTY_FUNCTION__ << ":" << a << endl;
}
int main()
{
int a = 6;
auto thread1 = std::thread(SimpleThread, a);
thread1.join();
return 0;
}
错误:
In file included from /usr/include/c++/4.8/thread:39:0,
from ./std_thread_refs.cpp:5:
/usr/include/c++/4.8/functional: In instantiation of ‘struct std::_Bind_simple<void (*(int))(int&)>’:
/usr/include/c++/4.8/thread:137:47: required from ‘std::thread::thread(_Callable&&, _Args&& ...) [with _Callable = void (&)(int&); _Args = {int&}]’
./std_thread_refs.cpp:19:47: required from here
/usr/include/c++/4.8/functional:1697:61: error: no type named ‘type’ in ‘class std::result_of<void (*(int))(int&)>’
typedef typename result_of<_Callable(_Args...)>::type result_type;
^
/usr/include/c++/4.8/functional:1727:9: error: no type named ‘type’ in ‘class std::result_of<void (*(int))(int&)>’
_M_invoke(_Index_tuple<_Indices...>)
^
我已改为传递指针,但有更好的解决方法吗?
I've changed to passing a pointer, but is there a better work around?
推荐答案
使用 reference_wrapper
使用 std::ref
:
Explicitly initialize the thread with a reference_wrapper
by using std::ref
:
auto thread1 = std::thread(SimpleThread, std::ref(a));
(或 std::cref
而不是 std::ref
,视情况而定).根据 cppreference on std:thread
的注释:
(or std::cref
instead of std::ref
, as appropriate). Per notes from cppreference on std:thread
:
线程函数的参数按值移动或复制.如果需要将引用参数传递给线程函数,则必须对其进行包装(例如使用 std::ref
或 std::cref
).
The arguments to the thread function are moved or copied by value. If a reference argument needs to be passed to the thread function, it has to be wrapped (e.g. with
std::ref
orstd::cref
).
这篇关于在 C++11 中通过引用 std::thread 传递对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 C++11 中通过引用 std::thread 传递对象
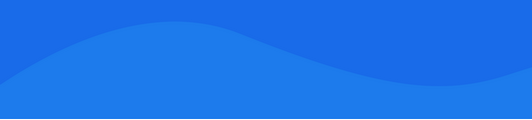
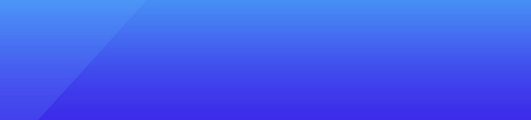
基础教程推荐
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 设计字符串本地化的最佳方法 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01