How to create a 2D array using a function?(如何使用函数创建二维数组?)
问题描述
我正在尝试定义一个二维数组,但我想在函数中进行,
I am trying to define a 2D array, but I want to do it in a function,
这是我的代码:
int** createArray( int columns, int rows)
{
int** array[rows];
for(int i = 0; i < rows; i++)
{
array[i] = new int*[columns];
}
for(int i = 0; i <columns; i++)
{
for(int j = 0; j < rows; j++)
{
array[i][j] = 0;
std::cout <<array[i][j];
}
std::cout<<"
";
}
return *array;
}
int main()
{
int **myArray = createArray(3,5);
for(int k =0; k < 5; k++)
{
if( (myArray[0][k] == 0) && (&myArray[1][k] == 0)) //segmentation fault
{
myArray[2][k] = 10; //segmentation fault
}
delete[] myArray;
}
但它会导致错误,可以看作是行中的注释.我是 C++ 新手,我不知道如何解决这个问题.
But it causes errors which can be seen as comments in lines. I am new to C++ and I do not know how to fix this.
非常感谢
推荐答案
std::vector
优于手动内存管理:
std::vector<std::vector<int>> createArray(int columns, int rows)
{
return std::vector<std::vector<int>(rows, std::vector<int>(columns));
}
int main()
{
int COLUMNS = 5;
int ROWS = 3;
auto myArray= createArray(COLUMNS, ROWS);
/*
Do stuff
*/
//std::vector handles delete on it's own, no need to clean up
}
如果由于某种原因不能使用 std::vector
,这是在堆上初始化 2D 数组的一种方法:
If you cannot use std::vector
for some reason, this is the a way to initialize 2D array on the heap:
int** createArray(int columns, int rows)
{
int** arr = new int*[rows];
for(int i = 0; i < rows; ++i)
{
arr[i] = new int[columns];
}
return arr;
}
int main()
{
int COLUMNS = 5;
int ROWS = 3;
int** myArray= createArray(COLUMNS, ROWS);
/*
Do stuff
*/
//you need to a delete for every new and delete[] for every new[]
for(int i = 0; i < rows; ++i)
{
delete[] myArray[i];
}
delete[] myArray;
}
这篇关于如何使用函数创建二维数组?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用函数创建二维数组?
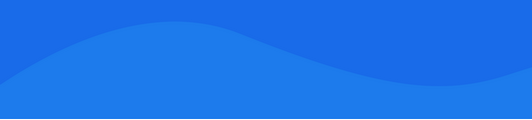
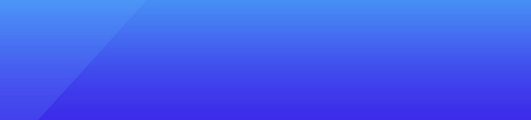
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 从 std::cin 读取密码 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01