C++ Update console output(C++ 更新控制台输出)
问题描述
I'm trying to make a program to print out a grid and given x and y co-ordinates change a value in the grid. For example, if the user entered X:0 and Y:0 it would change the value '9' in the image below to a predefined value (in this case I want to change the value 9 to 0).
My question is, is it possible to update the output of the console so that the '0' would override the '9' without printing out the entire grid again. I want to be able to do this multiple times.
If that is not possible, how can I print out the updated grid the way I have implemented this? If I were to put the display grid for loop in a separate function I would need to call the 2d array as a parameter which I'm sure you cannot do.
Here is what I have:
void generateGrid(int diff){
srand(time(NULL));
int arr[maximum][maximum];
for (int i=0;i<diff;i++)
{
for (int j=0;j<diff;j++)
{
arr[i][j] = rand() % 9 + 1;
}
}
cout<<"
Puzzle
";
for(int i=0;i<diff;i++)
{
cout<<i<<" ";
}
cout<<"
";
for(int i=0;i<diff;i++)
{
cout<<i<<" ";
for(int j=0;j<diff;j++)
{
cout<<arr[i][j]<<" ";
}
cout<<"
";
}
int x, y;
cout<<"
Enter x value: ";
cin>>x;
cout<<"Enter y value: ";
cin>>y;
arr[x][y] = 0;
}
Diff refers to the puzzle size (difficulty)
Elsewhere:
int easy = 5;
int medium = 8;
int hard = 10;
int maximum = 10;
Standard C++ does not support setting individual characters at positions in the console without re-printing. This is OS-specific, and there are comments that address this.
Otherwise, the correct solution is to encapsulate your game board logic into a class. We can use a nested std::vector
to handle a dynamically-sized board, and provide functions for getting and setting cells. A separate Print
function allows us to print the board to the console as often as we'd like.
class Grid
{
public:
Grid(int size) : myGrid(size, std::vector<int>(size, 0)) // initialize grid to be correctly sized and all zeros
{
Randomize();
}
void Randomize()
{
for (size_t i=0;i<myGrid.size();i++)
{
for (size_t j=0;j<myGrid[i].size();j++)
{
myGrid[i][j] = rand() % 9 + 1;
}
}
}
void Print(std::ostream& out) const
{
out<<"
Puzzle
";
for(size_t i=0;i<myGrid.size();i++)
{
out<<i<<" ";
}
out << "
";
for(size_t i=0;i<myGrid.size();i++)
{
out<<i<<" ";
for(size_t j=0;j<myGrid[i].size();j++)
{
out<<myGrid[i][j]<<" ";
}
out<<"
";
}
}
int GetValue(size_t row, size_t col) const
{
// use wraparound for too-large values
// alternatively you could throw if row and/or col are too large
return myGrid[row % myGrid.size()][col % myGrid.size()];
}
void SetValue(size_t row, size_t col, int val)
{
myGrid[row % myGrid.size()][col % myGrid.size()] = val;
}
private:
std::vector<std::vector<int>> myGrid;
};
Now you can write your main
like so:
int main()
{
srand(time(NULL));
Grid board(10);
size_t xValue = 0;
size_t yValue = 0;
// game loop. You could even abstract this behavior into another class
while(true)
{
board.Print(std::cout);
std::cout<<"
Enter x value: ";
if (!std::cin) // check for no input
break;
std::cin>>xValue;
if (!std::cin) // check for end of input
break;
std::cout<<"Enter y value: ";
std::cin>>yValue;
if (!std::cin)
break;
board.SetValue(xValue, yValue, 0);
// other game logic...
}
// print board one last time before exit
std::cout << "Game over. Final board:
";
board.Print(std::cout);
}
Live Demo
这篇关于C++ 更新控制台输出的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:C++ 更新控制台输出
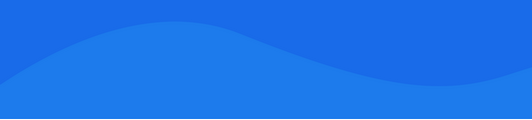
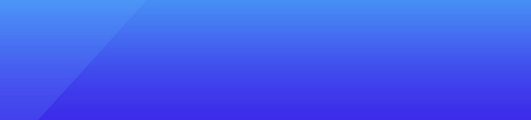
基础教程推荐
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01