From thrust::device_vector to raw pointer and back?(从推力::设备向量到原始指针并返回?)
问题描述
我了解如何从向量转到原始指针,但我跳过了关于如何倒退的节拍.
I understand how to go from a vector to a raw pointer but im skipping a beat on how to go backwards.
// our host vector
thrust::host_vector<dbl2> hVec;
// pretend we put data in it here
// get a device_vector
thrust::device_vector<dbl2> dVec = hVec;
// get the device ptr
thrust::device_ptr devPtr = &d_vec[0];
// now how do i get back to device_vector?
thrust::device_vector<dbl2> dVec2 = devPtr; // gives error
thrust::device_vector<dbl2> dVec2(devPtr); // gives error
有人可以解释/给我举个例子吗?
Can someone explain/point me to an example?
推荐答案
您像标准容器一样初始化和填充推力向量,即通过迭代器:
You initialize and populate thrust vectors just like standard containers, i.e. via iterators:
#include <thrust/device_vector.h>
#include <thrust/device_ptr.h>
int main()
{
thrust::device_vector<double> v1(10); // create a vector of size 10
thrust::device_ptr<double> dp = v1.data(); // or &v1[0]
thrust::device_vector<double> v2(v1); // from copy
thrust::device_vector<double> v3(dp, dp + 10); // from iterator range
thrust::device_vector<double> v4(v1.begin(), v1.end()); // from iterator range
}
在您的简单示例中,无需通过指针绕道,因为您可以直接复制另一个容器.一般来说,如果你有一个指向数组开头的指针,如果你提供数组大小,你可以使用 v3
的版本.
In your simple example there's no need to go the detour via pointers, as you can just copy the other container directly. In general, if you have a pointer to the beginning of an array, you can use the version for v3
if you supply the array size.
这篇关于从推力::设备向量到原始指针并返回?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从推力::设备向量到原始指针并返回?
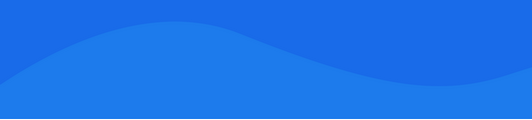
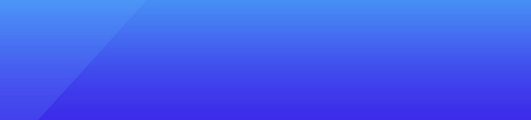
基础教程推荐
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01