g++ doesn#39;t compile constexpr function with assert in it(g++ 不会编译带有 assert 的 constexpr 函数)
问题描述
templateconstexpr 内联T getClamped(const T& mValue, const T& mMin, const T& mMax){断言(mMin < mMax);//删除这一行以成功编译返回 mValue <最小?mMin : (mValue > mMax ? mMax : mValue);}
<块引用>
错误: constexpr 函数体 'constexpr T getClamped(const T&, const T&, const T&) [with T = long unsigned int]' 不是返回语句
使用 g++ 4.8.1
.clang++ 3.4
不会抱怨.
谁在这里?有什么方法可以让 g++
在不使用宏的情况下编译代码?
GCC 是对的.但是,有一个相对简单的解决方法:
#include "assert.h"内联无效assert_helper(布尔测试){断言(测试);}内联 constexpr bool constexpr_assert( bool test ) {返回 test?true:(assert_helper(test),false);}模板<类型名 T>常量表达式inline T getClamped(const T& mValue, const T& mMin, const T& mMax){return constexpr_assert(mMin < mMax), (mValue < mMin ? mMin : (mValue > mMax ? mMax : mValue));}
我们两次滥用逗号操作符.
第一次是因为我们想要一个 assert
,当 true
时,可以从 constexpr
函数调用.第二个,所以我们可以将两个函数链接成一个 constexpr
函数.
作为附带的好处,如果 constexpr_assert
表达式在编译时无法验证为 true
,则 getClamped
函数不是 <代码>constexpr.
assert_helper
的存在是因为assert
的内容是NDEBUG
为真时定义的实现,所以我们不能把它嵌入到表达式中(它可以是语句,而不是表达式).它还保证失败的 constexpr_assert
失败是 constexpr
即使 assert
是 constexpr
(比如,当 NDEBUG
为假).
所有这一切的一个缺点是您的断言不是在发生问题的行触发,而是在更深的 2 个调用处触发.
template<typename T> constexpr inline
T getClamped(const T& mValue, const T& mMin, const T& mMax)
{
assert(mMin < mMax); // remove this line to successfully compile
return mValue < mMin ? mMin : (mValue > mMax ? mMax : mValue);
}
error: body of constexpr function 'constexpr T getClamped(const T&, const T&, const T&) [with T = long unsigned int]' not a return-statement
Using g++ 4.8.1
. clang++ 3.4
doesn't complain.
Who is right here? Any way I can make g++
compile the code without using macros?
GCC is right. However, there is a relatively simple workaround:
#include "assert.h"
inline void assert_helper( bool test ) {
assert(test);
}
inline constexpr bool constexpr_assert( bool test ) {
return test?true:(assert_helper(test),false);
}
template<typename T> constexpr
inline T getClamped(const T& mValue, const T& mMin, const T& mMax)
{
return constexpr_assert(mMin < mMax), (mValue < mMin ? mMin : (mValue > mMax ? mMax : mValue));
}
where we abuse the comma operator, twice.
The first time because we want to have an assert
that, when true
, can be called from a constexpr
function. The second, so we can chain two functions into a single constexpr
function.
As a side benefit, if the constexpr_assert
expression cannot be verified to be true
at compile time, then the getClamped
function is not constexpr
.
The assert_helper
exists because the contents of assert
are implementation defined when NDEBUG
is true, so we cannot embed it into an expression (it could be a statement, not an expression). It also guarantees that a failed constexpr_assert
fails to be constexpr
even if assert
is constexpr
(say, when NDEBUG
is false).
A downside to all of this is that your assert fires not at the line where the problem occurs, but 2 calls deeper.
这篇关于g++ 不会编译带有 assert 的 constexpr 函数的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:g++ 不会编译带有 assert 的 constexpr 函数
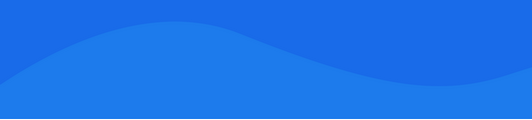
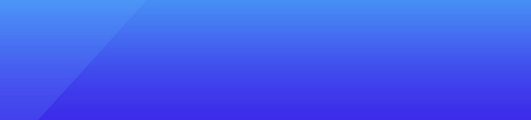
基础教程推荐
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 设计字符串本地化的最佳方法 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01