How to use va_list correctly in a sequence of wrapper functions calls?(如何在一系列包装函数调用中正确使用 va_list?)
问题描述
I have an ethernet library for microcontroller (keil rl-tcpnet for lpc2478). Library uses va_list (standard pointer to arg array macro defined in stdarg.h) in debug output function in such a way:
void __debug__ (const char *fmt, ...)
{
va_list args;
va_start (args,fmt);
vprintf (fmt,args);
va_end (args);
}
However vprintf sends data to incorrect stream and I need to redirect debug output of library to correct serial port. Library has .c cofigure files and I write c++ code so I use wrapper function pointers to call c++ style code from c code. So I rewrite this function:
extern void (* printDebugMsg)(const char * fmt, ...);
void __debug__ (const char *fmt, ...)
{
va_list args;
printDebugMsg(fmt, args);
}
other cpp file:
void printDebugMsgImplementation(const char * fmt, ...)
{
va_list args;
char buffer[100] = { 0 };
sprintf(buffer, fmt, args);
debugUart->write(buffer);
}
void (* printDebugMsg)(const char * fmt, ...) = &printDebugMsgImplementation;
The result debug output looks like:
TCP: Init 0 Sockets
IP : Src. IP : 0.0.14.1668436768
ETH: Dest.MAC: A1E05E10:A0002B44:5F9F:01:5FF8:33
The text and some numbers (like 0) seems to be correct but most other ones seems to be wrongly formatted (however it can be internal library problem also). I tried to rewrite my code using va_list as it is usually made in examples:
void __debug__ (const char *fmt, ...)
{
va_list args;
va_start (args,fmt);
printDebugMsg(fmt, args);
va_end (args);
}
and .cpp file:
void printDebugMsgImplementation(const char * fmt, ...)
{
va_list args;
char buffer[100] = { 0 };
va_start(args, fmt);
vsprintf(buffer, fmt, args);
va_end(args);
debugUart->write(buffer);
}
However the result is definetely worse that it was:
TCP: Init -1579131344 Sockets
ARP: Dest.IP: -1579131400.-1610601474.24805.4
ARP: Src.MAC: A1E05DF8:A0002BFE:60FF:04:616C:20
What I'm doing wrong? And how to deal with va_list in my case of wrapper functions calls?
You can't pass va_list
to a function expecting ...
, unless that function (of course) expects a va_list
in the variable-arguments part. Your code clearly does not.
You need to explicitly accept a va_list
argument into printDebugMsg()
, since that's what you're passing. See for instance vprintf()
which solves this problem for the printf()
family.
这篇关于如何在一系列包装函数调用中正确使用 va_list?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在一系列包装函数调用中正确使用 va_list?
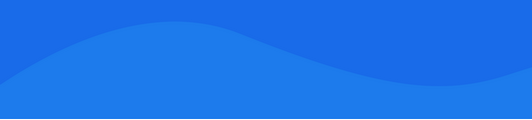
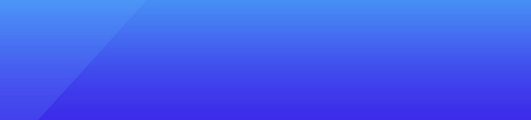
基础教程推荐
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17