Can I call a constructor from another constructor (do constructor chaining) in C++?(我可以从 C++ 中的另一个构造函数(做构造函数链接)调用构造函数吗?)
问题描述
作为 C# 开发人员,我习惯于通过构造函数运行:
As a C# developer I'm used to running through constructors:
class Test {
public Test() {
DoSomething();
}
public Test(int count) : this() {
DoSomethingWithCount(count);
}
public Test(int count, string name) : this(count) {
DoSomethingWithName(name);
}
}
有没有办法在 C++ 中做到这一点?
Is there a way to do this in C++?
我尝试调用类名并使用this"关键字,但都失败了.
I tried calling the Class name and using the 'this' keyword, but both fail.
推荐答案
C++11:是的!
C++11 及更高版本具有相同的功能(称为 委托构造函数).
C++11 and onwards has this same feature (called delegating constructors).
语法与C#略有不同:
class Foo {
public:
Foo(char x, int y) {}
Foo(int y) : Foo('a', y) {}
};
C++03:否
不幸的是,在 C++03 中没有办法做到这一点,但是有两种方法可以模拟这个:
Unfortunately, there's no way to do this in C++03, but there are two ways of simulating this:
您可以通过默认参数组合两个(或多个)构造函数:
You can combine two (or more) constructors via default parameters:
class Foo {
public:
Foo(char x, int y=0); // combines two constructors (char) and (char, int)
// ...
};
使用init方法共享通用代码:
Use an init method to share common code:
class Foo {
public:
Foo(char x);
Foo(char x, int y);
// ...
private:
void init(char x, int y);
};
Foo::Foo(char x)
{
init(x, int(x) + 7);
// ...
}
Foo::Foo(char x, int y)
{
init(x, y);
// ...
}
void Foo::init(char x, int y)
{
// ...
}
请参阅C++FAQ 条目以供参考.
这篇关于我可以从 C++ 中的另一个构造函数(做构造函数链接)调用构造函数吗?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:我可以从 C++ 中的另一个构造函数(做构造函数链接)调用构造函数吗?
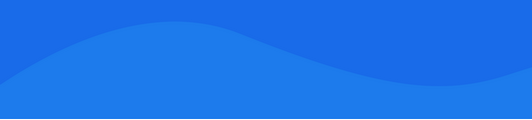
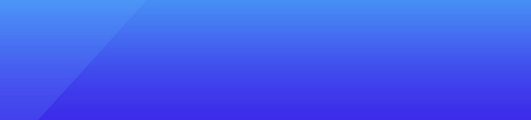
基础教程推荐
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- C++,'if' 表达式中的变量声明 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01