Thread pools not working with large number of tasks(线程池不能处理大量任务)
本文介绍了线程池不能处理大量任务的处理方法,对大家解决问题具有一定的参考价值,需要的朋友们下面随着小编来一起学习吧!
问题描述
我正在尝试使用原生C++创建一个线程池,并且我正在使用《操作中的C++并发》一书中的代码清单。我的问题是,当我提交的工作项比线程数多时,并不是所有的工作项都完成了。在下面的简单示例中,我试图提交RunMe()函数200次,但该函数只运行了8次。 这似乎不应该发生,因为在代码中,Work_Queue与工作线程是分开的。代码如下:
#include "iostream"
#include "ThreadPool.h"
void runMe()
{
cout << "testing" << endl;
}
int main(void)
{
thread_pool pool;
for (int i = 0; i < 200; i++)
{
std::function<void()> myFunction = [&] {runMe(); };
pool.submit(myFunction);
}
return 0;
}
ThreadPool.h类
#include <queue>
#include <future>
#include <list>
#include <functional>
#include <memory>
template<typename T>
class threadsafe_queue
{
private:
mutable std::mutex mut;
std::queue<T> data_queue;
std::condition_variable data_cond;
public:
threadsafe_queue() {}
void push(T new_value)
{
std::lock_guard<std::mutex> lk(mut);
data_queue.push(std::move(new_value));
data_cond.notify_one();
}
void wait_and_pop(T& value)
{
std::unique_lock<std::mutex> lk(mut);
data_cond.wait(lk, [this] {return !data_queue.empty(); });
value = std::move(data_queue.front());
data_queue.pop();
}
bool try_pop(T& value)
{
std::lock_guard<std::mutex> lk(mut);
if (data_queue.empty())
return false;
value = std::move(data_queue.front());
data_queue.pop();
return true;
}
bool empty() const
{
std::lock_guard<std::mutex> lk(mut);
return data_queue.empty();
}
int size()
{
return data_queue.size();
}
};
class join_threads
{
std::vector<std::thread>& threads;
public:
explicit join_threads(std::vector<std::thread>& threads_) : threads(threads_) {}
~join_threads()
{
for (unsigned long i = 0; i < threads.size(); i++)
{
if (threads[i].joinable())
{
threads[i].join();
}
}
}
};
class thread_pool
{
std::atomic_bool done;
threadsafe_queue<std::function<void()> > work_queue;
std::vector<std::thread> threads;
join_threads joiner;
void worker_thread()
{
while (!done)
{
std::function<void()> task;
if (work_queue.try_pop(task))
{
task();
numActiveThreads--;
}
else
{
std::this_thread::yield();
}
}
}
public:
int numActiveThreads;
thread_pool() : done(false), joiner(threads), numActiveThreads(0)
{
unsigned const thread_count = std::thread::hardware_concurrency();
try
{
for (unsigned i = 0; i < thread_count; i++)
{
threads.push_back(std::thread(&thread_pool::worker_thread, this));
}
}
catch (...)
{
done = true;
throw;
}
}
~thread_pool()
{
done = true;
}
template<typename FunctionType>
void submit(FunctionType f)
{
work_queue.push(std::function<void()>(f));
numActiveThreads++;
}
int size()
{
return work_queue.size();
}
bool isQueueEmpty()
{
return work_queue.empty();
}
};
关于如何正确使用Work_Queue有什么想法吗?
推荐答案
当pool
在main
结尾处被销毁时,您的析构函数集done
将使您的工作线程退出。
在设置标志之前,您应该使析构函数(如果要将其设置为可选,则可能使main
)等待队列排出。
这篇关于线程池不能处理大量任务的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
沃梦达教程
本文标题为:线程池不能处理大量任务
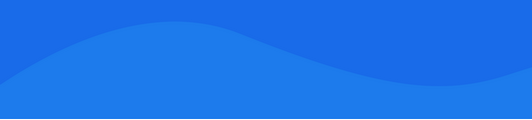
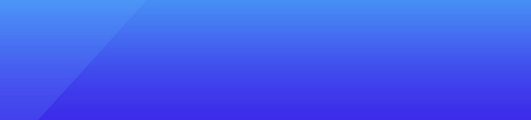
基础教程推荐
猜你喜欢
- 设计字符串本地化的最佳方法 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01