Stack overflow when debugging but not in release(调试时堆栈溢出,但不在版本中)
问题描述
我获得了以下代码,用于解析文本文件并对单词和行进行索引:
bool Database::addFromFileToListAndIndex(string path, BSTIndex* & index, list<Line *> & myList)
{
bool result = false;
ifstream txtFile;
txtFile.open(path, ifstream::in);
char line[200];
Line * ln;
//if path is valid AND is not already in the list then add it
if(txtFile.is_open() && (find(textFilePaths.begin(), textFilePaths.end(), path) == textFilePaths.end())) //the path is valid
{
//Add the path to the list of file paths
textFilePaths.push_back(path);
int lineNumber = 1;
while(!txtFile.eof())
{
txtFile.getline(line, 200);
ln = new Line(line, path, lineNumber);
if(ln->getLine() != "")
{
lineNumber++;
myList.push_back(ln);
vector<string> words = lineParser(ln);
for(unsigned int i = 0; i < words.size(); i++)
{
index->addWord(words[i], ln);
}
}
}
result = true;
}
return result;
}
我的代码运行得非常完美,速度也相当快,直到我给它一个巨大的文本文件。然后,我从Visual Studio收到堆栈溢出错误。当我切换到"Release"配置时,代码可以顺畅地运行。我的代码是否有问题,或者在运行"Debug"配置时是否存在某种限制?我是不是想在一个功能中做太多的事情?如果是,我如何分解它,使其在调试时不会崩溃?
编辑 根据请求,我实现了addWord;
void BSTIndex::addWord(BSTIndexNode *& pCurrentRoot, string word, Line * pLine)
{
if(pCurrentRoot == NULL) //BST is empty
{
BSTIndexNode * nodeToAdd = new BSTIndexNode();
nodeToAdd->word = word;
nodeToAdd->pData = pLine;
pCurrentRoot = nodeToAdd;
return;
}
//BST not empty
if (word < (pCurrentRoot->word)) //Go left
{
addWord(pCurrentRoot->pLeft, word, pLine);
}
else //Go right
{
addWord(pCurrentRoot->pRight, word, pLine);
}
}
和lineParser:
vector<string> Database::lineParser(Line * ln) //Parses a line and returns a vector of the words it contains
{
vector<string> result;
string word;
string line = ln->getLine();
//Regular Expression, matches anything that is not a letter, number, whitespace, or apostrophe
tr1::regex regEx("[^A-Za-z0-9\s\']");
//Using regEx above, replaces all non matching characters with nothing, essentially removing them.
line = tr1::regex_replace(line, regEx, std::string(""));
istringstream iss(line);
while(iss >> word)
{
word = getLowercaseWord(word);
result.push_back(word);
}
return result;
}
推荐答案
堆栈溢出表示堆栈空间已用完(可能很明显,但以防万一)。典型的原因是非终止或过度递归,或非常大的堆栈对象复制。有趣的是,在这种情况下,它可能是其中之一。
在发行版中,您的编译器很可能正在进行尾部调用优化,以防止过度递归导致堆栈溢出。
在发行版中,您的编译器也可能正在优化lineParser返回的向量副本。
因此您需要找出Debug中哪个条件溢出,我会从最有可能的罪魁祸首递归开始,尝试将字符串参数类型更改为引用,即。
void BSTIndex::addWord(BSTIndexNode *& pCurrentRoot, string & word, Line * pLine)
这应该可以防止您在每次嵌套调用addWord时复制Word对象。
还可以考虑将std::cout<;<;"Recursing addWord"<;<;std::Endl;type语句添加到addWord,这样您就可以看到它的深度以及它是否正确终止。
这篇关于调试时堆栈溢出,但不在版本中的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:调试时堆栈溢出,但不在版本中
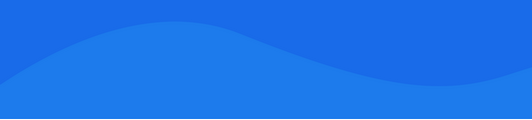
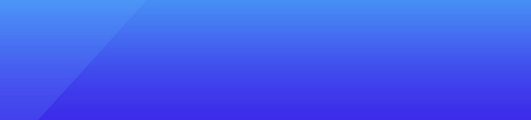
基础教程推荐
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 设计字符串本地化的最佳方法 2022-01-01