How to handle recursion in member functions?(如何处理成员函数中的递归?)
本文介绍了如何处理成员函数中的递归?的处理方法,对大家解决问题具有一定的参考价值,需要的朋友们下面随着小编来一起学习吧!
问题描述
例如,我有一个empty
函数来清除链表:
void empty(Node* head) {
if (head->next) { empty(head->next); }
delete head;
head = nullptr;
}
但后来我为链表创建了一个类,所以现在不需要传递head
参数:
void empty() {
if (head->next) { empty(head->next); }
delete head;
head = nullptr;
}
但是empty(head->next)
行显然是错误的,因为empty
不接受任何参数。我的想法是在函数中创建一个函数(使用lambda),类似于:
void empty() {
std::function<void(Node*)> emptyWrapper = [&] (Node* l_head) {
if (l_head->next) { emptyWrapper(l_head->next); }
delete l_head;
l_head = nullptr;
};
emptyWrapper(head);
}
但我想知道有没有更好的办法。最近,Lambdas对我来说是一种理想的固定方式。
推荐答案
一般方法是声明公共成员函数,该成员函数又调用私有静态递归成员函数。
请注意,名称empty
听起来令人困惑。最好将函数命名为clear
。
给您
#include <functional>
//...
class List
{
public:
//...
void clear()
{
clear( head );
}
private:
static void clear( Node * &head )
{
if ( head )
{
delete std::exchange( head, head->next );
clear( head );
}
}
//...
}
无需定义辅助静态函数即可使用相同的方法。
void clear()
{
if ( head )
{
delete std::exchange( head, head->next );
clear();
}
}
这里是一个演示程序。
#include <iostream>
#include <iomanip>
#include <functional>
template <typename T>
class List
{
private:
struct Node
{
T data;
Node *next;
} *head = nullptr;
public:
void push_front( const T &data )
{
head = new Node { data, head };
}
friend std::ostream & operator <<( std::ostream &os, const List &list )
{
for ( Node *current = list.head; current; current = current->next )
{
os << current->data << " -> ";
}
return os << "null";
}
bool empty() const { return head== nullptr; }
void clear()
{
if ( head )
{
delete std::exchange( head, head->next );
clear();
}
}
};
int main()
{
List<int> list;
const int N = 10;
for ( int i = N; i != 0; )
{
list.push_front( i-- );
}
std::cout << list << '
';
list.clear();
std::cout << "list is empty " << std::boolalpha << list.empty() << '
';
return 0;
}
程序输出为
1 -> 2 -> 3 -> 4 -> 5 -> 6 -> 7 -> 8 -> 9 -> 10 -> null
list is empty true
这篇关于如何处理成员函数中的递归?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
沃梦达教程
本文标题为:如何处理成员函数中的递归?
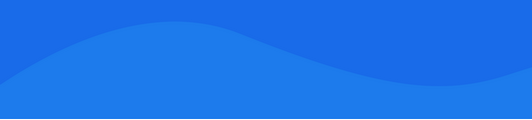
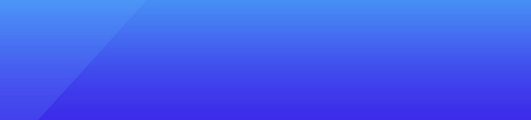
基础教程推荐
猜你喜欢
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 设计字符串本地化的最佳方法 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01