Most efficient way of reading data from a stream(从流中读取数据的最有效方式)
问题描述
我有一个使用对称加密来加密和解密数据的算法.无论如何,当我要解密时,我有:
I have an algorithm for encrypting and decrypting data using symmetric encryption. anyways when I am about to decrypt, I have:
CryptoStream cs = new CryptoStream(ms, cryptoTransform, CryptoStreamMode.Read);
我必须从 cs CryptoStream 读取数据并将该数据放入一个字节数组中.所以一种方法可能是:
I have to read data from the cs CryptoStream and place that data into a array of bytes. So one method could be:
System.Collections.Generic.List<byte> myListOfBytes = new System.Collections.Generic.List<byte>();
while (true)
{
int nextByte = cs.ReadByte();
if (nextByte == -1) break;
myListOfBytes.Add((Byte)nextByte);
}
return myListOfBytes.ToArray();
另一种技术可能是:
ArrayList chuncks = new ArrayList();
byte[] tempContainer = new byte[1048576];
int tempBytes = 0;
while (tempBytes < 1048576)
{
tempBytes = cs.Read(tempContainer, 0, tempContainer.Length);
//tempBytes is the number of bytes read from cs stream. those bytes are placed
// on the tempContainer array
chuncks.Add(tempContainer);
}
// later do a for each loop on chunks and add those bytes
我无法提前知道流 cs 的长度:
I cannot know in advance the length of the stream cs:
或者我应该实现我的堆栈类.我将加密很多信息,因此使此代码高效将节省大量时间
or perhaps I should implement my stack class. I will be encrypting a lot of information therefore making this code efficient will save a lot of time
推荐答案
你可以分块阅读:
using (var stream = new MemoryStream())
{
byte[] buffer = new byte[2048]; // read in chunks of 2KB
int bytesRead;
while((bytesRead = cs.Read(buffer, 0, buffer.Length)) > 0)
{
stream.Write(buffer, 0, bytesRead);
}
byte[] result = stream.ToArray();
// TODO: do something with the result
}
这篇关于从流中读取数据的最有效方式的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从流中读取数据的最有效方式
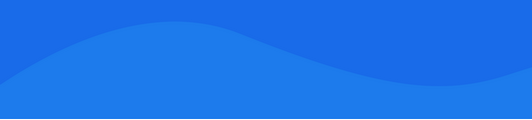
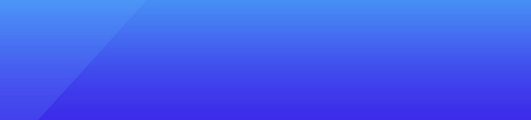
基础教程推荐
- 我什么时候应该使用 GC.SuppressFinalize()? 2022-01-01
- 覆盖 Json.Net 中的默认原始类型处理 2022-01-01
- C# - 将浮点数转换为整数...并根据余数更改整数 2022-01-01
- 如何使用OpenXML SDK将Excel转换为CSV? 2022-01-01
- 使用 SED 在 XML 标签之间提取值 2022-01-01
- Page.OnAppearing 中的 Xamarin.Forms Page.DisplayAlert 2022-01-01
- 从 VB6 迁移到 .NET/.NET Core 的最佳策略或工具 2022-01-01
- 当键值未知时反序列化 JSON 2022-01-01
- C# - 如何列出发布到 ASPX 页面的变量名称和值 2022-01-01
- 创建属性设置器委托 2022-01-01