How do I build a JSON object to send to an AJAX WebService?(如何构建 JSON 对象以发送到 AJAX WebService?)
问题描述
在尝试用 javascript 手动格式化我的 JSON 数据并惨遭失败后,我意识到可能有更好的方法.以下是 C# 中 Web 服务方法和相关类的代码:
After trying to format my JSON data by hand in javascript and failing miserably, I realized there's probably a better way. Here's what the code for the web service method and relevant classes looks like in C#:
[WebMethod]
public Response ValidateAddress(Request request)
{
return new test_AddressValidation().GenerateResponse(
test_AddressValidation.ResponseType.Ambiguous);
}
...
public class Request
{
public Address Address;
}
public class Address
{
public string Address1;
public string Address2;
public string City;
public string State;
public string Zip;
public AddressClassification AddressClassification;
}
public class AddressClassification
{
public int Code;
public string Description;
}
Web 服务非常适合使用 SOAP/XML,但我似乎无法使用 javascript 和 jQuery 获得有效响应,因为我从服务器返回的消息与我的手动编码 JSON 存在问题.
The web service works great with using SOAP/XML, but I can't seem to get a valid response using javascript and jQuery because the message I get back from the server has a problem with my hand-coded JSON.
我不能使用 jQuery getJSON
函数,因为请求需要 HTTP POST,所以我改用较低级别的 ajax
函数:
I can't use the jQuery getJSON
function because the request requires HTTP POST, so I'm using the lower-level ajax
function instead:
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
url: "http://bmccorm-xp/HBUpsAddressValidation/AddressValidation.asmx/ValidateAddress",
data: "{"Address":{"Address1":"123 Main Street","Address2":null,"City":"New York","State":"NY","Zip":"10000","AddressClassification":null}}",
dataType: "json",
success: function(response){
alert(response);
}
})
ajax
函数正在提交 data:
中指定的所有内容,这就是我的问题所在.如何在 javascript 中构建格式正确的 JSON 对象,以便可以将其插入到我的 ajax
调用中,如下所示:
The ajax
function is submitting everything specified in data:
, which is where my problem is. How do I build a properly formatted JSON object in javascript so I can plug it in to my ajax
call like so:
data: theRequest
我最终会从表单中的文本输入中提取数据,但目前硬编码的测试数据很好.
I'll eventually be pulling data out of text inputs in forms, but for now hard-coded test data is fine.
如何构建格式正确的 JSON 对象以发送到 Web 服务?
更新:事实证明,我的请求的问题不在于 JSON 的格式,因为 T.J.指出,而是我的 JSON 文本不符合 Web 服务的要求.这是基于 WebMethod 中的代码的有效 JSON 请求:
'{"request":{"Address":{"Address1":"123 Main Street","Address2":"suite 20","City":"New York","State":"NY","Zip":"10000","AddressClassification":null}}}'
这又引出了另一个问题:在对 ASP.NET Web 服务 (ASMX) 的 JSON 请求中,区分大小写何时重要?
This brought up another question: When is case sensitivity important in JSON requests to ASP.NET web services (ASMX)?
推荐答案
答案很简单,基于我之前的帖子 如果 ContentType 不是 JSON,我可以从 .asmx Web 服务返回 JSON 吗? 和 JQuery ajax 调用 httpget webmethod (c#) 不工作.
The answer is very easy and based on my previous posts Can I return JSON from an .asmx Web Service if the ContentType is not JSON? and JQuery ajax call to httpget webmethod (c#) not working.
数据应该是 JSON 编码的.您应该单独编码每个输入参数.因为你只有一个参数,你应该这样做:
The data should be JSON-encoded. You should separate encode every input parameter. Because you have only one parameter you should do like following:
首先将您的数据构建为原生 JavaScript 数据,例如:
first construct you data as native JavaScript data like:
var myData = {Address: {Address1:"address data 1",
Address2:"address data 2",
City: "Bonn",
State: "NRW",
Zip: "53353",
{Code: 123,
Description: "bla bla"}}};
然后作为ajax请求的参数给出{request:$.toJSON(myData)}
then give as a parameter of ajax request {request:$.toJSON(myData)}
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
url: "http://bmccorm-xp/HBUpsAddressValidation/AddressValidation.asmx/ValidateAddress",
data: {request:$.toJSON(myData)},
dataType: "json",
success: function(response){
alert(response);
}
})
您可以使用来自 http 的另一个版本 (JSON.stringify),而不是来自 JSON 插件的 $.toJSON://www.json.org/
如果你的 WebMethod 有类似的参数
If your WebMethod had parameters like
public Response ValidateAddress(Request request1, Request myRequest2)
ajax
调用的data
参数的值应该是这样的
the value of data
parameter of the ajax
call should be like
data: {request1:$.toJSON(myData1), myRequest2:$.toJSON(myData2)}
或
data: {request1:JSON.stringify(myData1), myRequest2:JSON.stringify(myData2)}
如果您更喜欢其他版本的 JSON 编码器.
if you prefer another version of JSON encoder.
这篇关于如何构建 JSON 对象以发送到 AJAX WebService?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何构建 JSON 对象以发送到 AJAX WebService?
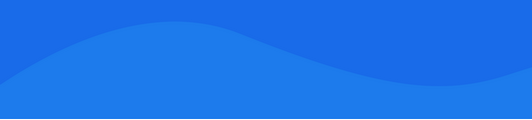
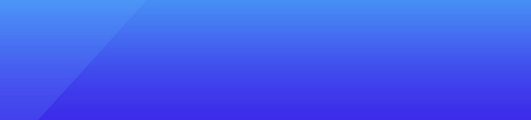
基础教程推荐
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- MS Visual Studio .NET 的替代品 2022-01-01
- 将 XML 转换为通用列表 2022-01-01