How to read embedded resource text file(如何读取嵌入式资源文本文件)
问题描述
如何使用 StreamReader
读取嵌入式资源(文本文件)并将其作为字符串返回?我当前的脚本使用 Windows 表单和文本框,允许用户在未嵌入的文本文件中查找和替换文本.
How do I read an embedded resource (text file) using StreamReader
and return it as a string? My current script uses a Windows form and textbox that allows the user to find and replace text in a text file that is not embedded.
private void button1_Click(object sender, EventArgs e)
{
StringCollection strValuesToSearch = new StringCollection();
strValuesToSearch.Add("Apple");
string stringToReplace;
stringToReplace = textBox1.Text;
StreamReader FileReader = new StreamReader(@"C:MyFile.txt");
string FileContents;
FileContents = FileReader.ReadToEnd();
FileReader.Close();
foreach (string s in strValuesToSearch)
{
if (FileContents.Contains(s))
FileContents = FileContents.Replace(s, stringToReplace);
}
StreamWriter FileWriter = new StreamWriter(@"MyFile.txt");
FileWriter.Write(FileContents);
FileWriter.Close();
}
推荐答案
您可以使用 Assembly.GetManifestResourceStream
方法:
添加以下用法
Add the following usings
using System.IO;
using System.Reflection;
设置相关文件的属性:
参数Build Action
,值为Embedded Resource
使用以下代码
var assembly = Assembly.GetExecutingAssembly();
var resourceName = "MyCompany.MyProduct.MyFile.txt";
using (Stream stream = assembly.GetManifestResourceStream(resourceName))
using (StreamReader reader = new StreamReader(stream))
{
string result = reader.ReadToEnd();
}
resourceName
是嵌入在 assembly
中的资源之一的名称.例如,如果您嵌入了一个名为 "MyFile.txt"
的文本文件,该文件位于具有默认命名空间 "MyCompany.MyProduct"
的项目的根目录中,则 >resourceName
是 "MyCompany.MyProduct.MyFile.txt"
.您可以使用 获取程序集中所有资源的列表Assembly.GetManifestResourceNames
方法.
resourceName
is the name of one of the resources embedded in assembly
.
For example, if you embed a text file named "MyFile.txt"
that is placed in the root of a project with default namespace "MyCompany.MyProduct"
, then resourceName
is "MyCompany.MyProduct.MyFile.txt"
.
You can get a list of all resources in an assembly using the Assembly.GetManifestResourceNames
Method.
<小时>
仅从文件名中获取 resourceName
的明智之举(通过命名空间的东西):
A no brainer astute to get the resourceName
from the file name only (by pass the namespace stuff):
string resourceName = assembly.GetManifestResourceNames()
.Single(str => str.EndsWith("YourFileName.txt"));
<小时>
一个完整的例子:
A complete example:
public string ReadResource(string name)
{
// Determine path
var assembly = Assembly.GetExecutingAssembly();
string resourcePath = name;
// Format: "{Namespace}.{Folder}.{filename}.{Extension}"
if (!name.StartsWith(nameof(SignificantDrawerCompiler)))
{
resourcePath = assembly.GetManifestResourceNames()
.Single(str => str.EndsWith(name));
}
using (Stream stream = assembly.GetManifestResourceStream(resourcePath))
using (StreamReader reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
这篇关于如何读取嵌入式资源文本文件的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何读取嵌入式资源文本文件
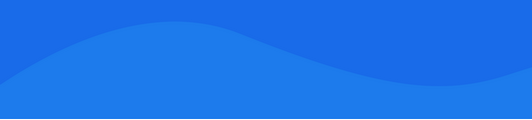
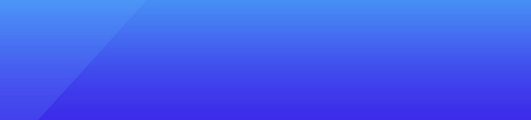
基础教程推荐
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- rabbitmq 的 REST API 2022-01-01