How to raise error if duplicates keys in dictionary(如果字典中的键重复,如何引发错误)
问题描述
如果用户在字典中输入重复键,我会尝试引发错误.字典在一个文件中,用户可以手动编辑该文件.
I try to raise an error if the user enter a duplicate key in a dictionary. The dictionary is in a file and the user can edit the file manually.
例子:
dico= {'root':{
'a':{'some_key':'value',...},
'b':{'some_key':'value',...},
'c':{'some_key':'value',...},
...
'a':{'some_key':'value',...},
}
}
新键a"已经存在...
the new key 'a' already exist...
当我从文件中加载 dico 时,如何测试 dico 并警告用户?
How can I test dico and warn the user when I load dico from the file?
推荐答案
写一个dict的子类,覆盖__setitem__,这样在替换现有key时会抛出错误;重写文件以使用新子类的构造函数而不是默认的 dict 内置函数.
Write a subclass of dict, override __setitem__ such that it throws an error when replacing an existing key; rewrite the file to use your new subclass's constructor instead of the default dict built-ins.
import collections
class Dict(dict):
def __init__(self, inp=None):
if isinstance(inp,dict):
super(Dict,self).__init__(inp)
else:
super(Dict,self).__init__()
if isinstance(inp, (collections.Mapping, collections.Iterable)):
si = self.__setitem__
for k,v in inp:
si(k,v)
def __setitem__(self, k, v):
try:
self.__getitem__(k)
raise ValueError("duplicate key '{0}' found".format(k))
except KeyError:
super(Dict,self).__setitem__(k,v)
那么你的文件必须写成
dico = Dict(
('root', Dict(
('a', Dict(
('some_key', 'value'),
('another_key', 'another_value')
),
('b', Dict(
('some_key', 'value')
),
('c', Dict(
('some_key', 'value'),
('another_key', 'another_value')
),
....
)
)
使用元组而不是字典来导入文件(使用 {} 表示法编写,它将使用默认的字典构造函数,并且重复项会在字典构造函数获取它们之前消失!).
using tuples instead of dicts for the file import (written using the {} notation, it would use the default dict constructor, and the duplicates would disappear before the Dict constructor ever gets them!).
这篇关于如果字典中的键重复,如何引发错误的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如果字典中的键重复,如何引发错误
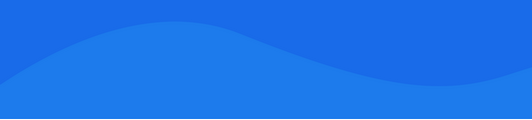
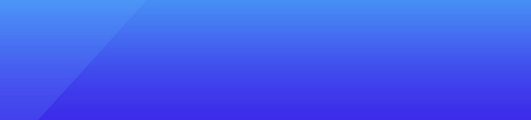
基础教程推荐
- Python 的 List 是如何实现的? 2022-01-01
- 如何在 Python 中检测文件是否为二进制(非文本)文 2022-01-01
- 使 Python 脚本在 Windows 上运行而不指定“.py";延期 2022-01-01
- 如何在Python中绘制多元函数? 2022-01-01
- 使用 Google App Engine (Python) 将文件上传到 Google Cloud Storage 2022-01-01
- 合并具有多索引的两个数据帧 2022-01-01
- 哪些 Python 包提供独立的事件系统? 2022-01-01
- 症状类型错误:无法确定关系的真值 2022-01-01
- 使用Python匹配Stata加权xtil命令的确定方法? 2022-01-01
- 将 YAML 文件转换为 python dict 2022-01-01