Convert binary string to bytearray in Python 3(在 Python 3 中将二进制字符串转换为字节数组)
问题描述
尽管有许多相关问题,但我找不到任何与我的问题相匹配的问题.我想将二进制字符串(例如,"0110100001101001"
)更改为字节数组(相同的示例,b"hi"
).
Despite the many related questions, I can't find any that match my problem. I'd like to change a binary string (for example, "0110100001101001"
) into a byte array (same example, b"hi"
).
我试过这个:
bytes([int(i) for i in "0110100001101001"])
但我得到了:
b'x00x01x01x00x01' #... and so on
在 Python 3 中执行此操作的正确方法是什么?
What's the correct way to do this in Python 3?
推荐答案
下面是 Patrick 提到的第一种方法的示例:将位串转换为 int 并一次取 8 位.这样做的自然方式以相反的顺序生成字节.为了让字节恢复到正确的顺序,我在字节数组上使用扩展切片表示法,步长为 -1:b[::-1]
.
Here's an example of doing it the first way that Patrick mentioned: convert the bitstring to an int and take 8 bits at a time. The natural way to do that generates the bytes in reverse order. To get the bytes back into the proper order I use extended slice notation on the bytearray with a step of -1: b[::-1]
.
def bitstring_to_bytes(s):
v = int(s, 2)
b = bytearray()
while v:
b.append(v & 0xff)
v >>= 8
return bytes(b[::-1])
s = "0110100001101001"
print(bitstring_to_bytes(s))
显然,Patrick 的第二种方式更为紧凑.:)
Clearly, Patrick's second way is more compact. :)
但是,在 Python 3 中有更好的方法来执行此操作:使用 int.to_bytes 方法:
However, there's a better way to do this in Python 3: use the int.to_bytes method:
def bitstring_to_bytes(s):
return int(s, 2).to_bytes((len(s) + 7) // 8, byteorder='big')
如果len(s)
保证是8的倍数,那么.to_bytes
的第一个arg可以简化:
If len(s)
is guaranteed to be a multiple of 8, then the first arg of .to_bytes
can be simplified:
return int(s, 2).to_bytes(len(s) // 8, byteorder='big')
如果 len(s)
不是 8 的倍数,这将引发 OverflowError
,这在某些情况下可能是可取的.
This will raise OverflowError
if len(s)
is not a multiple of 8, which may be desirable in some circumstances.
另一种选择是使用双重否定来执行天花板除法.对于整数 a &b、楼层划分使用//
Another option is to use double negation to perform ceiling division. For integers a & b, floor division using //
n = a // b
给出整数 n 使得
n <= a/b <n + 1
例如,47//10
给出 4,并且
gives the integer n such that
n <= a/b < n + 1
Eg,
47 // 10
gives 4, and
-47//10
给出 -5.所以
-(-47//10)
给出 5,有效地执行天花板除法.
-(-47 // 10)
gives 5, effectively performing ceiling division.
因此在 bitstring_to_bytes
我们可以 这样做:
Thus in bitstring_to_bytes
we could do:
return int(s, 2).to_bytes(-(-len(s) // 8), byteorder='big')
然而,熟悉这种高效 & 的人并不多.紧凑的成语,因此通常认为它的可读性不如
However, not many people are familiar with this efficient & compact idiom, so it's generally considered to be less readable than
return (s, 2).to_bytes((len(s) + 7) // 8, byteorder='big')
这篇关于在 Python 3 中将二进制字符串转换为字节数组的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 Python 3 中将二进制字符串转换为字节数组
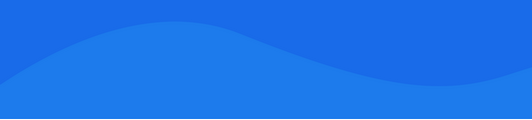
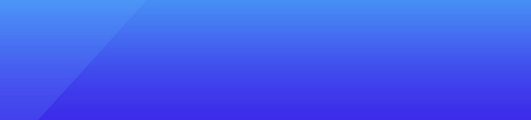
基础教程推荐
- 合并具有多索引的两个数据帧 2022-01-01
- 使用 Google App Engine (Python) 将文件上传到 Google Cloud Storage 2022-01-01
- 如何在Python中绘制多元函数? 2022-01-01
- 哪些 Python 包提供独立的事件系统? 2022-01-01
- 症状类型错误:无法确定关系的真值 2022-01-01
- 如何在 Python 中检测文件是否为二进制(非文本)文 2022-01-01
- 将 YAML 文件转换为 python dict 2022-01-01
- 使用Python匹配Stata加权xtil命令的确定方法? 2022-01-01
- Python 的 List 是如何实现的? 2022-01-01
- 使 Python 脚本在 Windows 上运行而不指定“.py";延期 2022-01-01