How to deal with certificates using Selenium?(如何使用 Selenium 处理证书?)
问题描述
I am using Selenium to launch a browser. How can I deal with the webpages (URLs) that will ask the browser to accept a certificate or not?
In Firefox, I may have a website like that asks me to accept its certificate like this:
On the Internet Explorer browser, I may get something like this:
On Google Chrome:
I repeat my question: How can I automate the acceptance of a website's certificate when I launch a browser (Internet Explorer, Firefox and Google Chrome) with Selenium (Python programming language)?
For the Firefox, you need to set accept_untrusted_certs
FirefoxProfile()
option to True
:
from selenium import webdriver
profile = webdriver.FirefoxProfile()
profile.accept_untrusted_certs = True
driver = webdriver.Firefox(firefox_profile=profile)
driver.get('https://cacert.org/')
driver.close()
For Chrome, you need to add --ignore-certificate-errors
ChromeOptions()
argument:
from selenium import webdriver
options = webdriver.ChromeOptions()
options.add_argument('ignore-certificate-errors')
driver = webdriver.Chrome(chrome_options=options)
driver.get('https://cacert.org/')
driver.close()
For the Internet Explorer, you need to set acceptSslCerts
desired capability:
from selenium import webdriver
capabilities = webdriver.DesiredCapabilities().INTERNETEXPLORER
capabilities['acceptSslCerts'] = True
driver = webdriver.Ie(capabilities=capabilities)
driver.get('https://cacert.org/')
driver.close()
Actually, according to the Desired Capabilities
documentation, setting acceptSslCerts
capability to True
should work for all browsers since it is a generic read/write capability:
acceptSslCerts
boolean
Whether the session should accept all SSL certs by default.
Working demo for Firefox:
>>> from selenium import webdriver
Setting acceptSslCerts
to False
:
>>> capabilities = webdriver.DesiredCapabilities().FIREFOX
>>> capabilities['acceptSslCerts'] = False
>>> driver = webdriver.Firefox(capabilities=capabilities)
>>> driver.get('https://cacert.org/')
>>> print(driver.title)
Untrusted Connection
>>> driver.close()
Setting acceptSslCerts
to True
:
>>> capabilities = webdriver.DesiredCapabilities().FIREFOX
>>> capabilities['acceptSslCerts'] = True
>>> driver = webdriver.Firefox(capabilities=capabilities)
>>> driver.get('https://cacert.org/')
>>> print(driver.title)
Welcome to CAcert.org
>>> driver.close()
这篇关于如何使用 Selenium 处理证书?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 Selenium 处理证书?
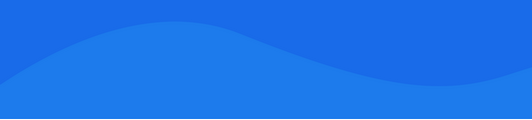
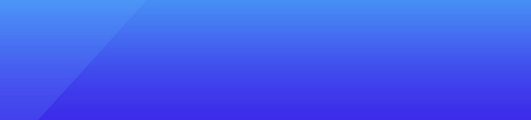
基础教程推荐
- 使用 Google App Engine (Python) 将文件上传到 Google Cloud Storage 2022-01-01
- 症状类型错误:无法确定关系的真值 2022-01-01
- 哪些 Python 包提供独立的事件系统? 2022-01-01
- 如何在Python中绘制多元函数? 2022-01-01
- 使用Python匹配Stata加权xtil命令的确定方法? 2022-01-01
- 如何在 Python 中检测文件是否为二进制(非文本)文 2022-01-01
- 将 YAML 文件转换为 python dict 2022-01-01
- 使 Python 脚本在 Windows 上运行而不指定“.py";延期 2022-01-01
- Python 的 List 是如何实现的? 2022-01-01
- 合并具有多索引的两个数据帧 2022-01-01