Remove elements of a vector inside the loop(删除循环内向量的元素)
问题描述
我知道有与这个类似的问题,但我没能在他们的帮助下找到我的代码的方法.我只想通过检查循环内此元素的属性来删除/删除向量的元素.我怎样才能做到这一点?我尝试了以下代码,但收到了模糊的错误消息:
I know that there are similar questions to this one, but I didn’t manage to find the way on my code by their aid. I want merely to delete/remove an element of a vector by checking an attribute of this element inside a loop. How can I do that? I tried the following code but I receive the vague message of error:
'operator =' 功能在播放器"中不可用.
'operator =' function is unavailable in 'Player’.
for (vector<Player>::iterator it = allPlayers.begin(); it != allPlayers.end(); it++)
{
if(it->getpMoney()<=0)
it = allPlayers.erase(it);
else
++it;
}
我该怎么办?
更新:你认为问题vector::erase with pointer成员属于同样的问题?因此我需要赋值运算符吗?为什么?
Update: Do you think that the question vector::erase with pointer member pertains to the same problem? Do I need hence an assignment operator? Why?
推荐答案
你不应该在 for
循环中增加 it
:
You should not increment it
in the for
loop:
for (vector<Player>::iterator it=allPlayers.begin();
it!=allPlayers.end();
/*it++*/) <----------- I commented it.
{
if(it->getpMoney()<=0)
it = allPlayers.erase(it);
else
++it;
}
注意注释部分;it++
在那里不需要,因为 it
在 for-body 本身中递增.
Notice the commented part;it++
is not needed there, as it
is getting incremented in the for-body itself.
至于'operator =' function isavailable in 'Player'"的错误,来自于内部使用的
移动向量中的元素.为了使用erase()
的使用operator=erase()
,Player
类的对象必须是可赋值的,这意味着你需要为实现
类.operator=
播放器
As for the error "'operator =' function is unavailable in 'Player’", it comes from the usage of erase()
which internally uses operator=
to move elements in the vector. In order to use erase()
, the objects of class Player
must be assignable, which means you need to implement operator=
for Player
class.
无论如何,您应该避免原始循环1 尽可能多,应该更喜欢使用算法.在这种情况下,流行的 Erase-Remove Idiom 可以简化您的操作正在做.
Anyway, you should avoid raw loop1 as much as possible and should prefer to use algorithms instead. In this case, the popular Erase-Remove Idiom can simplify what you're doing.
allPlayers.erase(
std::remove_if(
allPlayers.begin(),
allPlayers.end(),
[](Player const & p) { return p.getpMoney() <= 0; }
),
allPlayers.end()
);
1.这是我看过的Sean Parent 的最佳演讲之一.
这篇关于删除循环内向量的元素的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:删除循环内向量的元素
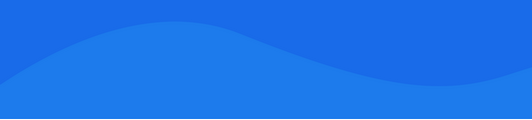
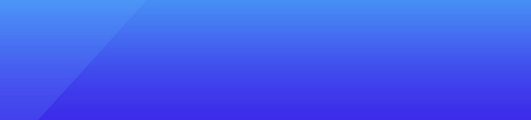
基础教程推荐
- 从 std::cin 读取密码 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01