How to draw a point (on mouseclick) on a QGraphicsScene?(如何在 QGraphicsScene 上绘制一个点(鼠标单击)?)
问题描述
我有以下代码来设置 QGraphicsScene
.我希望单击场景并在我单击的位置绘制一个点.我怎么能这样做?这是我当前的代码:
I have the following code to set up a QGraphicsScene
. I wish to click on the scene and draw a point at the location I've clicked. How could I do this? This is my current code:
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
QGraphicsScene *scene;
QGraphicsView *view = new QGraphicsView(this);
view->setGeometry(QRect(20, 50, 400, 400));
scene = new QGraphicsScene(50, 50, 350, 350);
view->setScene(scene);
}
推荐答案
UPDATE:有一个名为 QGraphicsSceneMouseEvent
的新类,它使这更容易一些.我刚刚在这里完成了一个使用它的例子:
UPDATE: There is a new class called QGraphicsSceneMouseEvent
that makes this a little easier.
I just finished an example using it here:
https://stackoverflow.com/a/26903599/999943
它与下面的答案的不同之处在于它子类化 QGraphicsScene
,而不是 QGraphicsView
,并且它使用 mouseEvent->scenePos()
所以无需手动映射坐标.
It differs with the answer below in that it subclasses QGraphicsScene
, not QGraphicsView
, and it uses mouseEvent->scenePos()
so there isn't a need to manually map coordinates.
您走在正确的轨道上,但您还有一些路要走.
You are on the right track, but you still have a little more to go.
您需要子类化 QGraphicsView
才能使用 QMouseEvent
通过鼠标按下或释放鼠标来执行某些操作.
You need to subclass QGraphicsView
to be able to do something with mouse presses or with mouse releases using QMouseEvent
.
#include <QGraphicsView>
#include <QGraphicsScene>
#include <QGraphicsEllipseItem>
#include <QMouseEvent>
class MyQGraphicsView : public QGraphicsView
{
Q_OBJECT
public:
explicit MyQGraphicsView(QWidget *parent = 0);
signals:
public slots:
void mousePressEvent(QMouseEvent * e);
// void mouseReleaseEvent(QMouseEvent * e);
// void mouseDoubleClickEvent(QMouseEvent * e);
// void mouseMoveEvent(QMouseEvent * e);
private:
QGraphicsScene * scene;
};
QGraphicsView
本身没有无量纲点.您可能希望使用 QGraphicsEllipse
项目或简单地使用半径非常小的 scene->addEllipseItem()
.
QGraphicsView
doesn't natively have dimension-less points. You will probably want to use QGraphicsEllipse
item or simply, scene->addEllipseItem()
with a very small radius.
#include "myqgraphicsview.h"
#include <QPointF>
MyQGraphicsView::MyQGraphicsView(QWidget *parent) :
QGraphicsView(parent)
{
scene = new QGraphicsScene();
this->setSceneRect(50, 50, 350, 350);
this->setScene(scene);
}
void MyQGraphicsView::mousePressEvent(QMouseEvent * e)
{
double rad = 1;
QPointF pt = mapToScene(e->pos());
scene->addEllipse(pt.x()-rad, pt.y()-rad, rad*2.0, rad*2.0,
QPen(), QBrush(Qt::SolidPattern));
}
注意 mapToScene()
的用法,使事件的 pos()
正确映射到鼠标在场景上点击的位置.
Note the usage of mapToScene()
to make the pos()
of the event map correctly to where the mouse is clicked on the scene.
如果要使用表单,则需要将子类 QGraphicsView
的实例添加到 ui 的 centralWidget 布局中.
You need to add an instance of your subclassed QGraphicsView
to the centralWidget's layout of your ui if you are going to use a form.
QGridLayout * gridLayout = new QGridLayout(ui->centralWidget);
gridLayout->addWidget( new MyQGraphicsView() );
或者如果您的 ui 已经有布局,它将如下所示:
or if your ui has a layout already it will look like this:
ui->centralWidget->layout()->addWidget( new MyGraphicsView() );
如果你不使用 QMainWindow
和一个表单,你可以将它添加到一个 QWidget
如果你为它设置一个布局然后添加你的 QGraphicsView
以类似的方式到该布局.如果您不想在 QGraphicsView
周围留出边距,只需调用 show 即可,不要将其放在不同的布局中.
If you don't use a QMainWindow
and a form, you can add it to a QWidget
if you set a layout for it and then add your QGraphicsView
to that layout in a similar manner. If you don't want a margin around your QGraphicsView
, just call show on it and don't put it inside a different layout.
#include <QtGui/QApplication>
#include "myqgraphicsview.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MyQGraphicsView view;
view.show();
return a.exec();
}
就是这样.现在您对 QGraphicsView
及其与鼠标的交互很危险.
And that's it. Now you are dangerous with QGraphicsView
's and their interaction with the mouse.
请务必阅读和研究 Qt 的图形视图框架和相关examples在使用QGraphicsView
和 QGraphicsScene
.它们是非常强大的 2D 图形工具,可能需要一些学习曲线,但它们值得.
Be sure to read and study about Qt's Graphics View Framework and the related examples to be effective when using QGraphicsView
and QGraphicsScene
. They are very powerful tools for 2D graphics and can have a bit of a learning curve but they are worth it.
这篇关于如何在 QGraphicsScene 上绘制一个点(鼠标单击)?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 QGraphicsScene 上绘制一个点(鼠标单击)?
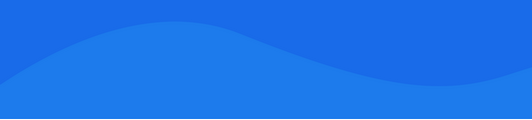
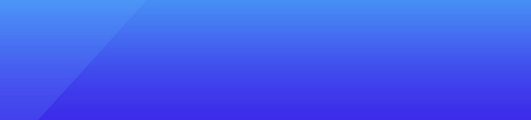
基础教程推荐
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 设计字符串本地化的最佳方法 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01