How can I loop through a C++ map of maps?(如何循环遍历地图的 C++ 地图?)
问题描述
如何在 C++ 中循环遍历 std::map
?我的地图定义为:
How can I loop through a std::map
in C++? My map is defined as:
std::map< std::string, std::map<std::string, std::string> >
例如上面的容器保存的数据是这样的:
For example, the above container holds data like this:
m["name1"]["value1"] = "data1";
m["name1"]["value2"] = "data2";
m["name2"]["value1"] = "data1";
m["name2"]["value2"] = "data2";
m["name3"]["value1"] = "data1";
m["name3"]["value2"] = "data2";
如何遍历此地图并访问各种值?
How can I loop through this map and access the various values?
推荐答案
老问题,但从 C++11 开始,其余答案已过时 - 您可以使用 范围基于 for 循环 只需执行:
Old question but the remaining answers are outdated as of C++11 - you can use a ranged based for loop and simply do:
std::map<std::string, std::map<std::string, std::string>> mymap;
for(auto const &ent1 : mymap) {
// ent1.first is the first key
for(auto const &ent2 : ent1.second) {
// ent2.first is the second key
// ent2.second is the data
}
}
这应该比早期版本更干净,并避免不必要的副本.
this should be much cleaner than the earlier versions, and avoids unnecessary copies.
有些人赞成用引用变量的显式定义替换注释(如果未使用,则会被优化掉):
Some favour replacing the comments with explicit definitions of reference variables (which get optimised away if unused):
for(auto const &ent1 : mymap) {
auto const &outer_key = ent1.first;
auto const &inner_map = ent1.second;
for(auto const &ent2 : inner_map) {
auto const &inner_key = ent2.first;
auto const &inner_value = ent2.second;
}
}
这篇关于如何循环遍历地图的 C++ 地图?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何循环遍历地图的 C++ 地图?
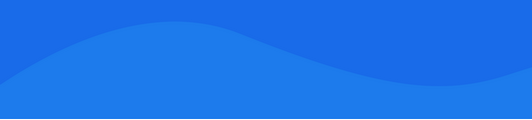
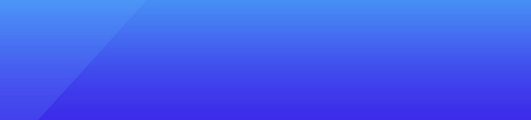
基础教程推荐
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 设计字符串本地化的最佳方法 2022-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01