iterate vector, remove certain items as I go(迭代向量,边走边删除某些项目)
问题描述
我有一个 std::vector m_vPaths;我将迭代这个向量并随时调用 ::DeleteFile(strPath) .如果我成功删除了文件,我会将其从向量中删除.我的问题是我可以避免使用两个向量吗?是否有不同的数据结构可能更适合我需要做的事情?
I have a std::vector m_vPaths; I will iterate this vector and call ::DeleteFile(strPath) as I go. If I successfully delete the file, I will remove it from the vector. My question is can I get around having to use two vectors? Is there different data structure that might be better suited for what I need to do?
示例:使用迭代器几乎可以满足我的要求,但问题是一旦使用迭代器擦除,所有迭代器都将无效.
example: using iterators almost does what I want, but problem is once you erase using an iterator, all iterators become invalid.
std::vector<std::string> iter = m_vPaths.begin();
for( ; iter != m_vPaths.end(); iter++) {
std::string strPath = *iter;
if(::DeleteFile(strPath.c_str())) {
m_vPaths.erase(iter);
//Now my interators are invalid because I used erase,
//but I want to continue deleteing the files remaining in my vector.
}
}
我可以使用两个向量并且不再有问题,但是有没有更好、更有效的方法来做我想做的事情?
I can use two vectors and I will no longer have a problem, but is there a better, more efficient method of doing what I'm trying to do?
顺便说一句,如果不清楚,m_vPaths 是这样声明的(在我的班级中):
btw, incase it is unclear, m_vPaths is declared like this (in my class):
std::vector<std::string> m_vPaths;
推荐答案
查看 std::remove_if
:
#include <algorithm> // for remove_if
#include <functional> // for unary_function
struct delete_file : public std::unary_function<const std::string&, bool>
{
bool operator()(const std::string& strPath) const
{
return ::DeleteFile(strPath.c_str());
}
}
m_vPaths.erase(std::remove_if(m_vPaths.begin(), m_vPaths.end(), delete_file()),
m_vPaths.end());
使用 std::list
停止无效迭代器问题,尽管您失去了随机访问.(和缓存性能,一般)
Use a std::list
to stop the invalid iterators problem, though you lose random access. (And cache performance, in general)
为了记录,您实现代码的方式是:
For the record, the way you would implement your code would be:
typedef std::vector<std::string> string_vector;
typedef std::vector<std::string>::iterator string_vector_iterator;
string_vector_iterator iter = m_vPaths.begin();
while (iter != m_vPaths.end())
{
if(::DeleteFile(iter->c_str()))
{
// erase returns the new iterator
iter = m_vPaths.erase(iter);
}
else
{
++iter;
}
}
但是你应该使用 std::remove_if
(重新发明轮子不好).
But you should use std::remove_if
(reinventing the wheel is bad).
这篇关于迭代向量,边走边删除某些项目的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:迭代向量,边走边删除某些项目
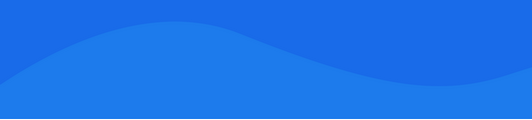
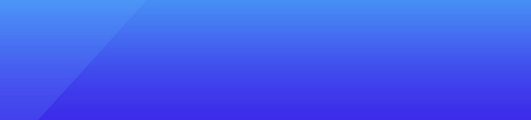
基础教程推荐
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- C++,'if' 表达式中的变量声明 2021-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 设计字符串本地化的最佳方法 2022-01-01